Write the following code using c #, Write a method with the following specifications: name: DisplayEquation arguments: none return value: nothing displays: the following table: x 5 3x -2x^2 y 0.0 5.0 0.0 0.0 5.0 0.3 5.0 0.8 -0.1 5.6 0.5 5.0 1.5 -0.5 6.0 0.8 5.0 2.3 -1.1 6.1 1.0 5.0 3.0 -2.0 6.0 1.3 5.0 3.8 -3.1 5.6 1.5 5.0 4.5 -4.5 5.0 1.8 5.0 5.3 -6.1 4.1 2.0 5.0 6.0 -8.0 3.0 This table is calculated using the quadratic equation y = 5 + 3x -2x2 The value of x starts at 0 and moves in increments of 0.25 to 2.0. (The first column is misleading because the last digit is rounded up i.e. 0.25 is displayed as 0.3and. 0.75 is displayed as 0.8) Modify your DisplayMenu method by adding another choice below the last item. In your main when the user enters the appropriate choice in response to the menu choices, you will invoke this method and to display the above values
时间: 2024-02-10 11:03:14 浏览: 119
Here's the C# code for the DisplayEquation method:
```csharp
public static void DisplayEquation()
{
Console.WriteLine("x\t5\t3x\t-2x^2\ty");
double x = 0.0;
while (x <= 2.0)
{
double y = 5 + 3 * x - 2 * x * x;
Console.WriteLine($"{x:f1}\t5.0\t{3*x:f1}\t{-2*x*x:f1}\t{y:f1}");
x += 0.25;
}
}
```
And here's an updated version of the DisplayMenu method that includes the new choice for displaying the equation table:
```csharp
public static void DisplayMenu()
{
Console.WriteLine("Choose an option:");
Console.WriteLine("1. Display Hello World");
Console.WriteLine("2. Display the current time");
Console.WriteLine("3. Display the quadratic equation table");
int choice = int.Parse(Console.ReadLine());
switch (choice)
{
case 1:
Console.WriteLine("Hello World!");
break;
case 2:
Console.WriteLine($"The current time is {DateTime.Now}");
break;
case 3:
DisplayEquation();
break;
default:
Console.WriteLine("Invalid choice");
break;
}
}
```
To call the DisplayEquation method, you can simply add a new case to the switch statement in your main method:
```csharp
while (true)
{
DisplayMenu();
Console.WriteLine("Press any key to continue...");
Console.ReadKey();
}
```
And when the user enters "3" at the menu, the quadratic equation table will be displayed.
阅读全文
相关推荐
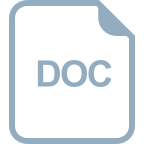
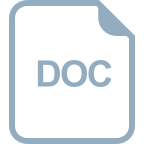
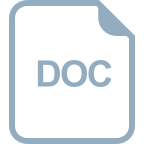
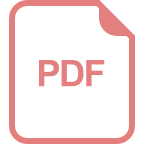
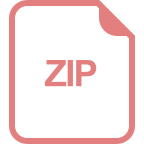
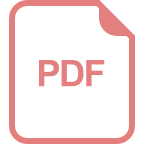
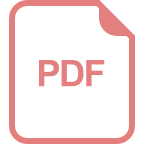
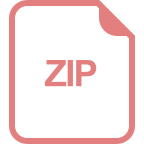
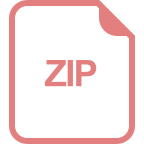
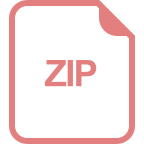
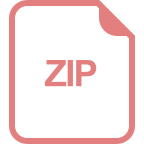
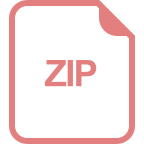
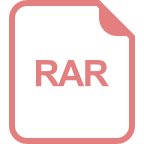
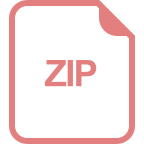
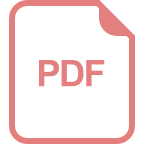
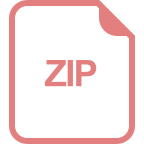
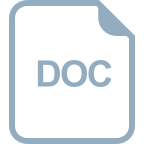
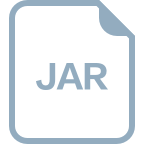