请继续完善使用qt libvncclient库来实现一个vnc客户端协议
时间: 2024-05-12 13:13:31 浏览: 15
接下来,我们可以使用Qt的网络模块和libvncclient库来实现一个VNC客户端协议。
首先,我们需要在Qt项目中添加libvncclient库。在.pro文件中添加以下内容:
```
LIBS += -lvncclient
```
接下来,我们需要创建一个VNC客户端类,并在其中实现连接远程VNC服务器、发送鼠标和键盘事件等方法。
```c++
#include <QObject>
#include <QImage>
#include <QSize>
#include <QPoint>
#include <rfb/rfbclient.h>
class VncClient : public QObject
{
Q_OBJECT
public:
explicit VncClient(QObject *parent = nullptr);
~VncClient();
void connectToServer(QString address, QString password);
void disconnectFromServer();
void sendMouseEvent(QPoint pos, Qt::MouseButton button, bool press);
void sendKeyEvent(int key, bool press);
signals:
void connected();
void disconnected();
void authenticationFailed();
void authenticationSucceeded();
void updateScreen(QImage image, QSize size);
private:
rfbClient* m_client;
QImage m_image;
QSize m_size;
static void updateScreenCallback(rfbClient* client, int x, int y, int w, int h);
};
```
在VncClient类中,我们使用rfbClient结构体来连接远程VNC服务器。rfbClient是libvncclient库中的结构体,用于存储VNC客户端的状态信息。
```c++
m_client = rfbGetClient(8, 3, 4);
m_client->serverHost = strdup(address.toUtf8().data());
m_client->passwd = strdup(password.toUtf8().data());
m_client->MallocFrameBuffer = nullptr;
m_client->serverPort = 5900;
m_client->listenPort = 0;
m_client->shared = true;
m_client->frameBuffer = nullptr;
m_client->frameBufferDepth = 0;
m_client->frameBufferFormat = nullptr;
rfbSetLogDest(RFB_LOG_NONE);
rfbInitClient(m_client, nullptr, nullptr);
```
在连接到服务器后,我们可以使用rfbClient结构体中的回调函数来处理VNC服务器发送的屏幕更新数据。在updateScreenCallback()回调函数中,我们使用QImage对象来存储屏幕上的像素数据,并通过信号发送给主线程。
```c++
void VncClient::updateScreenCallback(rfbClient* client, int x, int y, int w, int h)
{
auto self = reinterpret_cast<VncClient*>(client->clientData);
self->m_image = QImage(client->frameBuffer + y * client->width * (client->format.bitsPerPixel / 8),
w, h, QImage::Format_RGB32);
self->m_size = QSize(client->width, client->height);
emit self->updateScreen(self->m_image, self->m_size);
}
```
我们还需要实现发送鼠标和键盘事件的方法。在sendMouseEvent()方法中,我们使用rfbClient结构体中的函数来模拟鼠标事件。在sendKeyEvent()方法中,我们使用rfbClient结构体中的函数来模拟键盘事件。
```c++
void VncClient::sendMouseEvent(QPoint pos, Qt::MouseButton button, bool press)
{
int buttonMask = (button == Qt::LeftButton ? rfbButton1Mask :
(button == Qt::RightButton ? rfbButton3Mask : rfbButton2Mask));
rfbClientSendPointerEvent(m_client, pos.x(), pos.y(), buttonMask, press);
}
void VncClient::sendKeyEvent(int key, bool press)
{
rfbClientSendKeyEvent(m_client, key, press);
}
```
最后,在VncClient类的析构函数中,我们需要释放rfbClient结构体和其他资源。
```c++
VncClient::~VncClient()
{
rfbClientCleanup(m_client);
free(m_client);
m_client = nullptr;
free(m_image.bits());
m_image = QImage();
}
```
现在我们已经实现了一个基本的VNC客户端,可以连接到远程VNC服务器并显示屏幕数据。我们可以在主窗口中创建一个QGraphicsView控件来显示屏幕数据,并通过VncClient类中的信号来更新它的内容。
```c++
void MainWindow::onUpdateScreen(QImage image, QSize size)
{
m_scene->clear();
auto pixmap = QPixmap::fromImage(image);
auto item = new QGraphicsPixmapItem(pixmap);
m_scene->addItem(item);
m_view->setSceneRect(0, 0, size.width(), size.height());
m_view->fitInView(item, Qt::KeepAspectRatio);
}
```
最后,我们可以在主窗口中添加一些按钮和文本框,以便用户输入远程VNC服务器的地址和密码,并连接到它。我们还可以在按钮的clicked()信号中调用VncClient类中的方法来发送鼠标和键盘事件。
完整的示例代码见下:
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QGraphicsView>
#include <QGraphicsScene>
#include <QLineEdit>
#include <QPushButton>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QKeyEvent>
#include "vncclient.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
, m_scene(new QGraphicsScene(this))
, m_view(new QGraphicsView(m_scene, this))
, m_addressInput(new QLineEdit(this))
, m_passwordInput(new QLineEdit(this))
, m_connectButton(new QPushButton(tr("Connect"), this))
, m_disconnectButton(new QPushButton(tr("Disconnect"), this))
, m_client(new VncClient(this))
{
ui->setupUi(this);
m_view->setRenderHint(QPainter::Antialiasing);
m_view->setRenderHint(QPainter::SmoothPixmapTransform);
m_view->setDragMode(QGraphicsView::ScrollHandDrag);
m_view->setViewportUpdateMode(QGraphicsView::FullViewportUpdate);
m_view->setOptimizationFlag(QGraphicsView::DontAdjustForAntialiasing, true);
m_view->setOptimizationFlag(QGraphicsView::DontSavePainterState, true);
m_view->setOptimizationFlag(QGraphicsView::DontAdjustForAntialiasing, true);
m_view->setOptimizationFlag(QGraphicsView::DontAdjustForAntialiasing, true);
m_view->setHorizontalScrollBarPolicy(Qt::ScrollBarAlwaysOff);
m_view->setVerticalScrollBarPolicy(Qt::ScrollBarAlwaysOff);
m_view->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Expanding);
auto inputLayout = new QHBoxLayout();
inputLayout->addWidget(new QLabel(tr("Address:"), this));
inputLayout->addWidget(m_addressInput);
inputLayout->addWidget(new QLabel(tr("Password:"), this));
inputLayout->addWidget(m_passwordInput);
auto buttonLayout = new QHBoxLayout();
buttonLayout->addWidget(m_connectButton);
buttonLayout->addWidget(m_disconnectButton);
auto mainLayout = new QVBoxLayout();
mainLayout->addWidget(m_view);
mainLayout->addLayout(inputLayout);
mainLayout->addLayout(buttonLayout);
auto centralWidget = new QWidget(this);
centralWidget->setLayout(mainLayout);
setCentralWidget(centralWidget);
connect(m_connectButton, &QPushButton::clicked, this, &MainWindow::onConnectButtonClicked);
connect(m_disconnectButton, &QPushButton::clicked, this, &MainWindow::onDisconnectButtonClicked);
connect(m_client, &VncClient::connected, this, &MainWindow::onConnected);
connect(m_client, &VncClient::disconnected, this, &MainWindow::onDisconnected);
connect(m_client, &VncClient::authenticationFailed, this, &MainWindow::onAuthenticationFailed);
connect(m_client, &VncClient::authenticationSucceeded, this, &MainWindow::onAuthenticationSucceeded);
connect(m_client, &VncClient::updateScreen, this, &MainWindow::onUpdateScreen);
setMinimumSize(800, 600);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::keyPressEvent(QKeyEvent *event)
{
m_client->sendKeyEvent(event->key(), true);
}
void MainWindow::keyReleaseEvent(QKeyEvent *event)
{
m_client->sendKeyEvent(event->key(), false);
}
void MainWindow::onConnectButtonClicked()
{
m_client->connectToServer(m_addressInput->text(), m_passwordInput->text());
}
void MainWindow::onDisconnectButtonClicked()
{
m_client->disconnectFromServer();
}
void MainWindow::onConnected()
{
m_connectButton->setEnabled(false);
m_disconnectButton->setEnabled(true);
}
void MainWindow::onDisconnected()
{
m_connectButton->setEnabled(true);
m_disconnectButton->setEnabled(false);
}
void MainWindow::onAuthenticationFailed()
{
qDebug() << "Authentication failed";
}
void MainWindow::onAuthenticationSucceeded()
{
qDebug() << "Authentication succeeded";
}
void MainWindow::onUpdateScreen(QImage image, QSize size)
{
m_scene->clear();
auto pixmap = QPixmap::fromImage(image);
auto item = new QGraphicsPixmapItem(pixmap);
m_scene->addItem(item);
m_view->setSceneRect(0, 0, size.width(), size.height());
m_view->fitInView(item, Qt::KeepAspectRatio);
}
```
这就是使用Qt和libvncclient库实现VNC客户端协议的基本步骤。通过这个例子,我们可以学习如何使用第三方C++库,并将其与Qt的其他模块结合起来实现一个完整的应用程序。
相关推荐
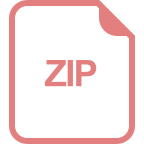
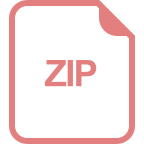














