python 在已知等高线图中寻找同心等高线圈
时间: 2024-03-15 16:45:06 浏览: 140
要在已知等高线图中寻找同心等高线圈,可以使用 Python 中的 OpenCV 库和 NumPy 库。
以下是一个示例代码,用于在一个等高线图像中找到同心圆:
```python
import cv2
import numpy as np
# 读取等高线图像
img = cv2.imread('contour.png', 0)
# 阈值化处理
ret, thresh = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY)
# 膨胀操作
kernel = np.ones((5, 5), np.uint8)
dilation = cv2.dilate(thresh, kernel, iterations=2)
# 检测轮廓
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 绘制同心圆
for i in range(1, 6):
# 计算同心圆的半径
radius = i * 10
# 创建一个空白图像
circle_img = np.zeros_like(img)
# 绘制同心圆
cv2.circle(circle_img, (img.shape[1]//2, img.shape[0]//2), radius, 255, 1)
# 计算同心圆与等高线的交集
intersection = cv2.bitwise_and(circle_img, thresh)
# 绘制交集
cv2.drawContours(intersection, contours, -1, 255, thickness=1)
# 显示结果
cv2.imshow('Intersection of circle and contours', intersection)
cv2.waitKey()
# 关闭窗口
cv2.destroyAllWindows()
```
在这个示例代码中,我们首先读取了一个等高线图像,然后使用阈值化和膨胀操作来检测轮廓。接着,我们使用 `cv2.circle` 函数绘制了 5 个同心圆,并且计算了同心圆与等高线的交集。最后,我们使用 `cv2.drawContours` 函数将交集绘制出来,并且使用 `cv2.imshow` 函数显示结果。
注意,这个示例代码中的等高线图像必须是单通道的灰度图像。如果你的等高线图像是彩色的,需要首先将其转换为灰度图像。
阅读全文
相关推荐
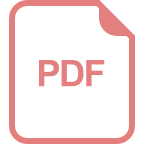
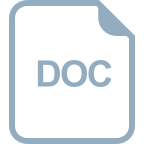
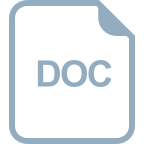













