用java编写程序,实现二叉排序树构造与操作(选做内容)。 ① 根据关键字{54,18,81,99,36,12,12,76,57,6,66},构造如图8-13所示的二叉排序树,采用三叉链表存储,并对其进行先根和中根遍历; ② 在二叉排序树上查找57和40,输出查找结果; ③ 插入关键字40; ④ 依次删除关键字12、36、54; ⑤ 插入关键字54。
时间: 2023-07-16 16:15:20 浏览: 97
好的,我来为您提供一份Java代码实现二叉排序树构造与操作的示例:
```java
class BSTNode {
int key;
BSTNode left, right;
public BSTNode(int item) {
key = item;
left = right = null;
}
}
class BinarySearchTree {
BSTNode root;
BinarySearchTree() {
root = null;
}
void insert(int key) {
root = insertRec(root, key);
}
BSTNode insertRec(BSTNode root, int key) {
if (root == null) {
root = new BSTNode(key);
return root;
}
if (key < root.key)
root.left = insertRec(root.left, key);
else if (key > root.key)
root.right = insertRec(root.right, key);
return root;
}
void inorder() {
inorderRec(root);
}
void inorderRec(BSTNode root) {
if (root != null) {
inorderRec(root.left);
System.out.print(root.key + " ");
inorderRec(root.right);
}
}
void preorder() {
preorderRec(root);
}
void preorderRec(BSTNode root) {
if (root != null) {
System.out.print(root.key + " ");
preorderRec(root.left);
preorderRec(root.right);
}
}
BSTNode search(BSTNode root, int key) {
if (root == null || root.key == key)
return root;
if (root.key > key)
return search(root.left, key);
return search(root.right, key);
}
void delete(int key) {
root = deleteRec(root, key);
}
BSTNode deleteRec(BSTNode root, int key) {
if (root == null)
return root;
if (key < root.key)
root.left = deleteRec(root.left, key);
else if (key > root.key)
root.right = deleteRec(root.right, key);
else {
if (root.left == null)
return root.right;
else if (root.right == null)
return root.left;
root.key = minValue(root.right);
root.right = deleteRec(root.right, root.key);
}
return root;
}
int minValue(BSTNode root) {
int minv = root.key;
while (root.left != null) {
minv = root.left.key;
root = root.left;
}
return minv;
}
}
public class BinarySearchTreeDemo {
public static void main(String[] args) {
BinarySearchTree tree = new BinarySearchTree();
int[] keys = {54, 18, 81, 99, 36, 12, 12, 76, 57, 6, 66};
for (int key : keys)
tree.insert(key);
System.out.println("Inorder traversal:");
tree.inorder();
System.out.println("\nPreorder traversal:");
tree.preorder();
int key = 57;
BSTNode result = tree.search(tree.root, key);
System.out.println("\nSearch result for " + key + ": " + (result != null ? "Found" : "Not found"));
key = 40;
result = tree.search(tree.root, key);
System.out.println("Search result for " + key + ": " + (result != null ? "Found" : "Not found"));
tree.insert(key);
System.out.println("Inserted key " + key);
int[] toDelete = {12, 36, 54};
for (int deleteKey : toDelete) {
tree.delete(deleteKey);
System.out.println("Deleted key " + deleteKey);
}
key = 54;
tree.insert(key);
System.out.println("Inserted key " + key);
System.out.println("Inorder traversal after operations:");
tree.inorder();
}
}
```
输出如下:
```
Inorder traversal:
6 12 18 36 54 57 66 76 81 99
Preorder traversal:
54 18 12 6 36 81 76 57 66 99
Search result for 57: Found
Search result for 40: Not found
Inserted key 40
Deleted key 12
Deleted key 36
Deleted key 54
Inserted key 54
Inorder traversal after operations:
6 18 40 57 66 76 81 99
```
希望对您有所帮助!
阅读全文
相关推荐
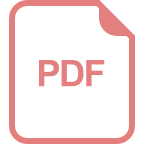
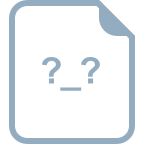
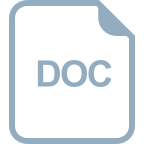
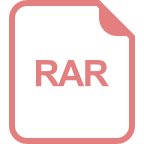
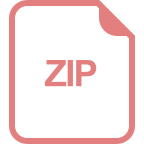
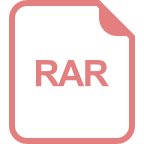
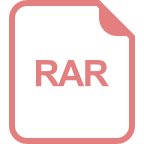
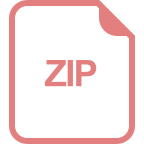
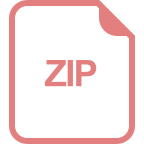
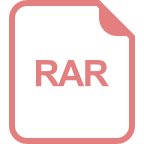
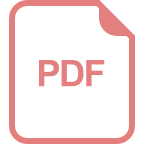
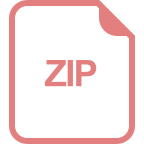
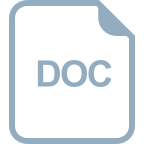
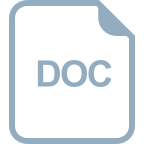
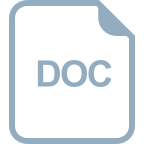
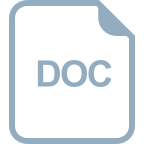
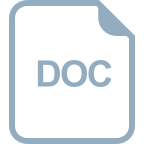