首页java语言编写程序,可以输入一个中缀表达式,并将其转化为后缀表达式,并计算出结果
java语言编写程序,可以输入一个中缀表达式,并将其转化为后缀表达式,并计算出结果
时间: 2024-05-26 11:10:08 浏览: 62
你好,我可以回答这个问题。JAVA语言编写程序可以实现将中缀表达式转化为后缀表达式,并计算出结果。中缀表达式是常见的数学表达式,例如 3 + 5 * 2 - 6 / 3。而后缀表达式,也叫逆波兰表达式,是将操作符放在数字之后的表达式,例如 3 5 2 * + 6 3 / -。关于如何编写这样的程序,可以参考算法书籍或者相关的编程教程。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
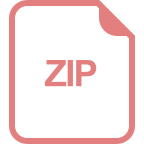
基于Flask,mysql slope one的图书推荐系统全部资料+详细文档.zip
【资源说明】
基于Flask,mysql slope one的图书推荐系统全部资料+详细文档.zip
【备注】
1、该项目是个人高分项目源码,已获导师指导认可通过,答辩评审分达到95分
2、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用!
3、本项目适合计算机相关专业(人工智能、通信工程、自动化、电子信息、物联网等)的在校学生、老师或者企业员工下载使用,也可作为毕业设计、课程设计、作业、项目初期立项演示等,当然也适合小白学习进阶。
4、如果基础还行,可以在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。
欢迎下载,沟通交流,互相学习,共同进步!
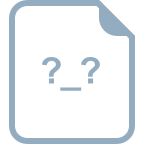
舰艇2 glb模型文件,航空母舰glb模型(亲测可用) 效果图见描述
可用于cesium、threejs等模型文件。
https://i-blog.csdnimg.cn/direct/e7a6309fedca4d4f93bdac4b12f6545e.png
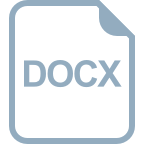
HengCe-18900-2024-2030中国聚乙烯醇缩丁醛市场现状研究分析与发展前景预测报告-样本.docx
HengCe-18900-2024-2030中国聚乙烯醇缩丁醛市场现状研究分析与发展前景预测报告-样本.docx
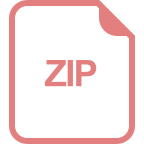
SpringBoot集成Neo4j图数据库+朴素贝叶斯分类器实现基于电影知识图谱的智能问答系统源码+项目说明.zip
本资源是一套基于SpringBoot、Neo4j图数据库及朴素贝叶斯分类器的电影知识图谱智能问答系统源码与项目说明。通过集成Neo4j,实现电影数据的高效存储和查询;利用Spark的MLlib库中的朴素贝叶斯分类器,对用户问答进行智能处理和分类。该系统不仅展示了如何构建复杂的数据处理流程,还提供了详细的配置文件和项目文档。此资源适合有一定编程基础且希望深入理解智能问答系统开发的学习者使用,能够帮助掌握SpringBoot、Neo4j以及机器学习在实际项目中的应用。请注意,该资源为学习资源,不包含具体安装步骤和详细部署指南。
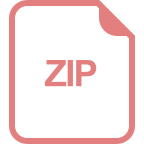
GTK 的 Go 绑定.zip
转到gtk 什么是GTK 的 Go 绑定截屏安装您可以通过运行各种示例程序来试验 go-gtkgit clone https://github.com/mattn/go-gtkcd go-gtkgo get ...make example./example/demo/demo或者go get github.com/mattn/go-gtk/gtk不要忘记,您需要 GTK-Development-Packages。如果您使用 Linux,则应该安装libgtk+-2.0依赖于 gtk 的软件包。在 Debian 和 Debian 衍生发行版上,您可以运行(以 root 身份)apt-get install libgtk2.0-dev libglib2.0-dev libgtksourceview2.0-dev如果你使用 Windows,请从这里找到 gtk 二进制包https://www.gtk.org/docs/installations/windows嵌入可以使用以下方式嵌入 pixbuf 图像$ g
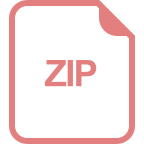
WordPress作为新闻管理面板的实现指南
资源摘要信息: "使用WordPress作为管理面板"
WordPress,作为当今最流行的开源内容管理系统(CMS),除了用于搭建网站、博客外,还可以作为一个功能强大的后台管理面板。本示例展示了如何利用WordPress的后端功能来管理新闻或帖子,将WordPress用作组织和发布内容的管理面板。
首先,需要了解WordPress的基本架构,包括它的数据库结构和如何通过主题和插件进行扩展。WordPress的核心功能已经包括文章(帖子)、页面、评论、分类和标签的管理,这些都可以通过其自带的仪表板进行管理。
在本示例中,WordPress被用作一个独立的后台管理面板来管理新闻或帖子。这种方法的好处是,WordPress的用户界面(UI)友好且功能全面,能够帮助不熟悉技术的用户轻松管理内容。WordPress的主题系统允许用户更改外观,而插件架构则可以扩展额外的功能,比如表单生成、数据分析等。
实施该方法的步骤可能包括:
1. 安装WordPress:按照标准流程在指定目录下安装WordPress。
2. 数据库配置:需要修改WordPress的配置文件(wp-config.php),将数据库连接信息替换为当前系统的数据库信息。
3. 插件选择与定制:可能需要安装特定插件来增强内容管理的功能,或者对现有的插件进行定制以满足特定需求。
4. 主题定制:选择一个适合的WordPress主题或者对现有主题进行定制,以实现所需的视觉和布局效果。
5. 后端访问安全:由于将WordPress用于管理面板,需要考虑安全性设置,如设置强密码、使用安全插件等。
值得一提的是,虽然WordPress已经内置了丰富的管理功能,但在企业级应用中,还需要考虑性能优化、安全性增强、用户权限管理等方面。此外,由于WordPress主要是作为内容发布平台设计的,将其作为管理面板可能需要一定的定制工作以确保满足特定的业务需求。
【PHP】标签意味着在实现该示例时,需要使用PHP编程语言。WordPress本身是由PHP语言开发的,因此开发者可能需要具备PHP开发能力,或至少能够理解PHP代码基础,以便对WordPress进行定制和扩展。
最后,【压缩包子文件的文件名称列表】中的"dctb-wp-as-admin-panel-master"暗示了这是一个存放所有相关文件的主目录,包括源代码、配置文件、模板文件、资源文件等,这些文件是安装和运行WordPress管理面板所必需的。在实际部署时,开发者需要上传这些文件到服务器,按照文档说明进行配置和安装。
总之,本示例提供了一个通过WordPress来搭建一个简单新闻或帖子管理系统的思路,展示了如何利用现有的开源工具来实现特定的业务需求。开发者需要有相应的PHP和WordPress知识,以及对网络安全和性能优化的基本了解。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
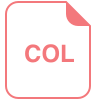
函数与模块化编程宝典:J750编程高效之路
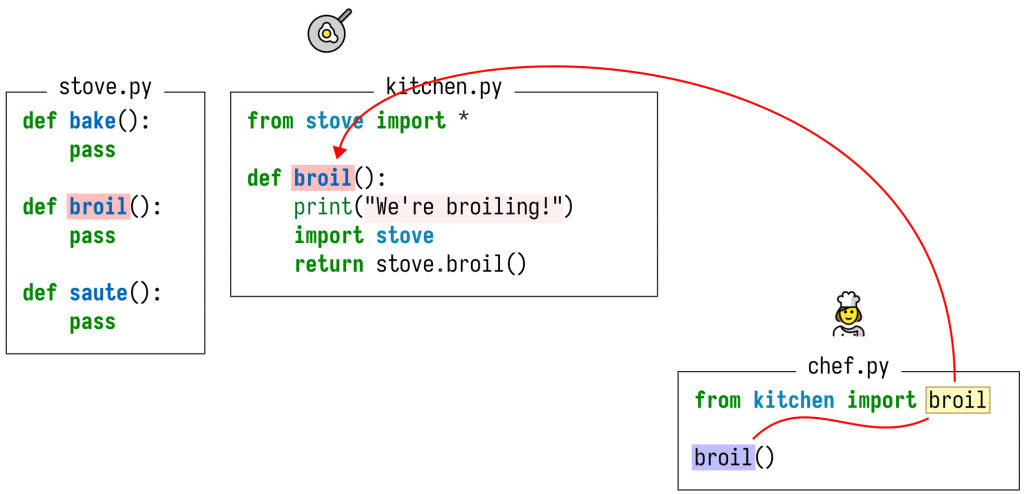
参考资源链接:[泰瑞达J750设备编程基础教程](https://wenku.csdn.net/doc/6412b472be7fbd1778d3f9e1?spm=1055.2635.3001.10343)
# 1. 函数与模块化编程基础
## 1.1 编程中的函数基础
函数是编程的基础构件,它允许将代码块组织成可重复使用的组件。在函数中封装特定的逻辑

用C语言求有4个圆塔,圆心分别为(2,2),(2,-2),(-2,2),(-2,-2)圆半径为1, 这4个塔的高度为10m 塔以外无建筑物接输入任意点的坐标 求该点的建筑高度(塔外的高度为零)的程序
在C语言中,你可以编写一个简单的函数来解决这个问题。首先,你需要确定每个圆是否包含了给定的点。如果包含,则返回塔高10米,如果不包含则返回0。这里提供一个基本的伪代码思路:
```c
#include <stdio.h>
#include <math.h>
// 定义圆的结构体
typedef struct {
double x, y; // 圆心坐标
int radius; // 半径
} Circle;
// 函数判断点是否在圆内
int is_point_in_circle(Circle circle, double px, double py) {
d
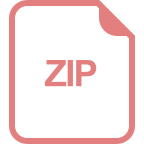
NPC_Generator:使用Ruby打造的游戏角色生成器
资源摘要信息:"NPC_Generator是一个专门为角色扮演游戏(RPG)或模拟类游戏设计的角色生成工具,它允许游戏开发者或者爱好者快速创建非玩家角色(NPC)并赋予它们丰富的背景故事、外观特征以及可能的行为模式。NPC_Generator的开发使用了Ruby编程语言,Ruby以其简洁的语法和强大的编程能力在脚本编写和小型项目开发中十分受欢迎。利用Ruby编写的NPC_Generator可以集成到游戏开发流程中,实现自动化生成NPC,极大地节省了手动设计每个NPC的时间和精力,提升了游戏内容的丰富性和多样性。"
知识点详细说明:
1. NPC_Generator的用途:
NPC_Generator是用于游戏角色生成的工具,它能够帮助游戏设计师和玩家创建大量的非玩家角色(Non-Player Characters,简称NPC)。在RPG或模拟类游戏中,NPC是指在游戏中由计算机控制的虚拟角色,它们与玩家角色互动,为游戏世界增添真实感。
2. NPC生成的关键要素:
- 角色背景故事:每个NPC都应该有自己的故事背景,这些故事可以是关于它们的过去,它们为什么会在游戏中出现,以及它们的个性和动机等。
- 外观特征:NPC的外观包括性别、年龄、种族、服装、发型等,这些特征可以由工具随机生成或者由设计师自定义。
- 行为模式:NPC的行为模式决定了它们在游戏中的行为方式,比如友好、中立或敌对,以及它们可能会执行的任务或对话。
3. Ruby编程语言的优势:
- 简洁的语法:Ruby语言的语法非常接近英语,使得编写和阅读代码都变得更加容易和直观。
- 灵活性和表达性:Ruby语言提供的大量内置函数和库使得开发者可以快速实现复杂的功能。
- 开源和社区支持:Ruby是一个开源项目,有着庞大的开发者社区和丰富的学习资源,有利于项目的开发和维护。
4. 项目集成与自动化:
NPC_Generator的自动化特性意味着它可以与游戏引擎或开发环境集成,为游戏提供即时的角色生成服务。自动化不仅可以提高生成NPC的效率,还可以确保游戏中每个NPC都具备独特的特性,使游戏世界更加多元和真实。
5. 游戏开发的影响:
NPC_Generator的引入对游戏开发产生以下影响:
- 提高效率:通过自动化的角色生成,游戏开发团队可以节约大量时间和资源,专注于游戏设计的其他方面。
- 增加多样性:自动化的工具可以根据不同的参数生成大量不同的NPC,为游戏世界带来更多的故事线和交互可能性。
- 玩家体验:丰富的NPC角色能够提升玩家的沉浸感,使得玩家在游戏中的体验更加真实和有吸引力。
6. Ruby在游戏开发中的应用:
虽然Ruby不是游戏开发中最常用的编程语言,但其在小型项目、原型设计、脚本编写等领域有其独特的优势。一些游戏开发工具和框架支持Ruby,如Ruby on Rails可以在Web游戏开发中发挥作用,而一些游戏开发社区也在探索Ruby的更多潜力。
7. NPC_Generator的扩展性和维护:
为了确保NPC_Generator能够长期有效地工作,它需要具备良好的扩展性和维护性。这意味着工具应该支持插件或模块的添加,允许社区贡献新功能,并且代码应该易于阅读和修改,以便于未来的升级和优化。
综上所述,NPC_Generator是一款利用Ruby编程语言开发的高效角色生成工具,它不仅提高了游戏开发的效率,而且通过提供丰富多样的NPC角色增加了游戏的深度和吸引力。随着游戏开发的不断发展,此类自动化工具将变得更加重要,而Ruby作为一种支持快速开发的编程语言,在这一领域有着重要的应用前景。