txt文件里的文本内容根据指定的分割字符数目区间进行随机换行切分,python类实现
时间: 2023-05-25 09:05:34 浏览: 120
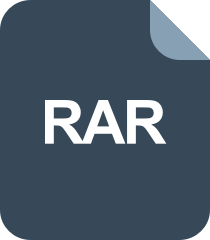
Python中文文本分析(期末大作业).rar

以下是一个Python类,可将给定的文本文件内容根据指定的分割字符数目区间进行随机换行切分:
```python
import random
class TxtLineRandomSplitter:
def __init__(self, filename, minchars, maxchars):
self.filename = filename
self.minchars = minchars
self.maxchars = maxchars
def readlines(self):
with open(self.filename, 'r', encoding='utf-8') as f:
return f.readlines()
def generate_random_line_splits(self, line):
splits = []
start = 0
while start < len(line):
end = start + random.randint(self.minchars, self.maxchars)
if end >= len(line):
end = len(line) - 1
while end > start and line[end] != ' ':
end -= 1
splits.append(line[start:end])
start = end + 1
return splits
def split_lines(self):
lines = self.readlines()
split_lines = []
for line in lines:
split_lines += self.generate_random_line_splits(line)
return split_lines
```
上面的类 `TxtLineRandomSplitter` 接受三个参数:
- `filename`:文本文件的文件名,需要是一个字符串
- `minchars`:最小的字符数目,用于随机决定每行的分割位置
- `maxchars`:最大的字符数目,用于随机决定每行的分割位置
使用该类的方式如下:
```python
splitter = TxtLineRandomSplitter('example.txt', 50, 70)
split_lines = splitter.split_lines()
for line in split_lines:
print(line)
```
其中,`example.txt` 是要分割的文本文件,`50` 和 `70` 分别是分割字符数目区间的最小值和最大值。该类会读取 `example.txt` 中的所有行,将各行随机分割成多个子行,并将所有子行存储在一个列表中 `split_lines`,最后逐行输出所有子行。
阅读全文
相关推荐
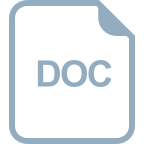
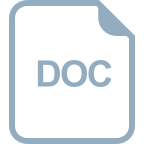
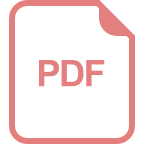
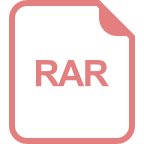
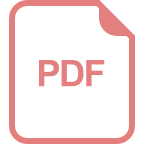
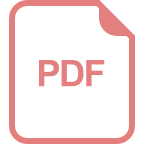
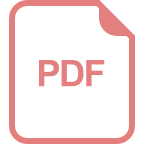
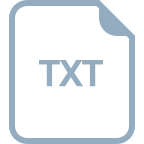
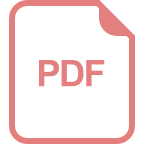
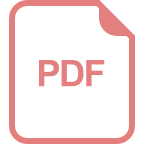
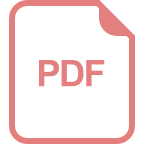
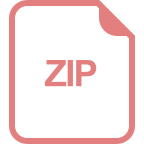
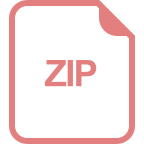
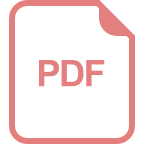
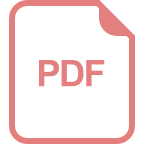
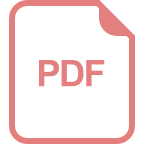