c++实现以点(point)类为基类,派生圆类。圆由圆心和半径定义。派生类操作判断任一坐标点是在图形内,还是在图形的边缘上,还是在图形外。
时间: 2024-02-25 13:59:10 浏览: 21
好的,这是一个比较典型的面向对象编程问题。下面是一个简单的 C++ 实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Point {
public:
Point(double x=0, double y=0): x(x), y(y) {}
double getX() { return x; }
double getY() { return y; }
private:
double x, y;
};
class Circle: public Point {
public:
Circle(double x=0, double y=0, double r=0): Point(x, y), r(r) {}
double getR() { return r; }
bool contains(Point &p) {
double dx = p.getX() - getX();
double dy = p.getY() - getY();
double distance = sqrt(dx * dx + dy * dy);
return distance < r;
}
bool onBoundary(Point &p) {
double dx = p.getX() - getX();
double dy = p.getY() - getY();
double distance = sqrt(dx * dx + dy * dy);
return abs(distance - r) < 1e-6;
}
private:
double r;
};
int main() {
Circle c(0, 0, 5);
Point p1(3, 4);
Point p2(5, 0);
Point p3(6, 0);
if (c.contains(p1)) {
cout << "p1 is inside the circle." << endl;
}
if (c.onBoundary(p2)) {
cout << "p2 is on the boundary of the circle." << endl;
}
if (!c.contains(p3)) {
cout << "p3 is outside the circle." << endl;
}
return 0;
}
```
这个实现中,我们定义了 `Point` 类和 `Circle` 类。`Point` 类表示一个点,具有 `x` 和 `y` 坐标;`Circle` 类继承自 `Point` 类,表示一个圆,具有圆心坐标和半径。在 `Circle` 类中,我们实现了两个方法:`contains` 和 `onBoundary`。`contains` 方法用于判断任意一个点是否在圆内;`onBoundary` 方法用于判断任意一个点是否在圆的边缘上。
在主函数中,我们创建了一个圆 `c`,并分别判断了三个点 `p1`、`p2`、`p3` 是否在圆内、在圆的边缘上、在圆外,分别输出了相应的结果。
相关推荐
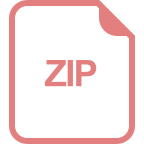
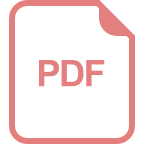
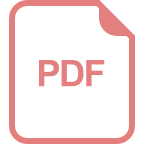














