用java得GUI写一个登陆界面
时间: 2023-05-30 22:07:36 浏览: 129
以下是一个基本的Java GUI登录界面的代码示例:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class LoginGUI extends JFrame implements ActionListener {
private JTextField usernameField;
private JPasswordField passwordField;
public LoginGUI() {
super("Login");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridLayout(3, 2));
JLabel usernameLabel = new JLabel("Username:");
panel.add(usernameLabel);
usernameField = new JTextField(20);
panel.add(usernameField);
JLabel passwordLabel = new JLabel("Password:");
panel.add(passwordLabel);
passwordField = new JPasswordField(20);
panel.add(passwordField);
JButton loginButton = new JButton("Login");
loginButton.addActionListener(this);
panel.add(loginButton);
JButton cancelButton = new JButton("Cancel");
cancelButton.addActionListener(this);
panel.add(cancelButton);
add(panel);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
if (command.equals("Login")) {
String username = usernameField.getText();
String password = new String(passwordField.getPassword());
if (username.equals("admin") && password.equals("password")) {
JOptionPane.showMessageDialog(this, "Login Successful");
} else {
JOptionPane.showMessageDialog(this, "Invalid Username or Password");
}
} else if (command.equals("Cancel")) {
System.exit(0);
}
}
public static void main(String[] args) {
new LoginGUI();
}
}
```
在这个示例中,我们使用了JFrame作为窗口容器,JPanel作为主面板,GridLayout来布局,JLabel来显示标签,JTextField和JPasswordField用于输入用户名和密码,JButton用于提交和取消登录。我们还实现了ActionListener接口,以便处理登录和取消按钮的点击事件。在登录按钮被点击时,我们检查用户名和密码是否为"admin"和"password",如果是则显示登录成功的消息框,否则显示无效的用户名或密码的消息框。在取消按钮被点击时,我们关闭窗口并退出应用程序。
阅读全文
相关推荐



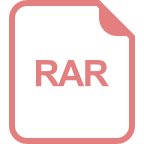
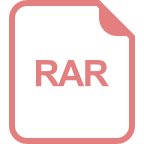



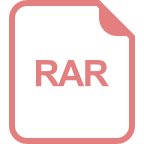



