ffmpeg6 解码rtsp视频流 c++
时间: 2023-10-24 12:02:49 浏览: 253
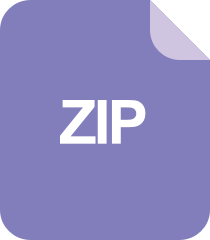
ffmpeg_rtsp_mpp:ffmpeg 拉取rtsp h264流, 使用mpp解码, 目前在firefly 板子上跑通了

使用 C++ 解码 RTSP 视频流需要使用 FFmpeg 库。以下是一个简单的示例代码:
```
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
}
int main()
{
av_register_all();
avformat_network_init();
AVFormatContext* pFormatCtx = NULL;
AVCodecContext* pCodecCtx = NULL;
AVCodec* pCodec = NULL;
AVPacket packet;
AVFrame* pFrame = NULL;
const char* url = "rtsp://example.com/stream";
if (avformat_open_input(&pFormatCtx, url, NULL, NULL) != 0)
{
return -1;
}
if (avformat_find_stream_info(pFormatCtx, NULL) < 0)
{
return -1;
}
int videoStream = -1;
for (int i = 0; i < pFormatCtx->nb_streams; i++)
{
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO)
{
videoStream = i;
break;
}
}
if (videoStream == -1)
{
return -1;
}
pCodecCtx = avcodec_alloc_context3(NULL);
avcodec_parameters_to_context(pCodecCtx, pFormatCtx->streams[videoStream]->codecpar);
pCodec = avcodec_find_decoder(pCodecCtx->codec_id);
if (pCodec == NULL)
{
return -1;
}
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0)
{
return -1;
}
pFrame = av_frame_alloc();
while (av_read_frame(pFormatCtx, &packet) >= 0)
{
if (packet.stream_index == videoStream)
{
if (avcodec_send_packet(pCodecCtx, &packet) < 0)
{
break;
}
while (avcodec_receive_frame(pCodecCtx, pFrame) == 0)
{
// 处理解码后的图像数据
}
}
av_packet_unref(&packet);
}
avformat_close_input(&pFormatCtx);
avcodec_free_context(&pCodecCtx);
av_frame_free(&pFrame);
return 0;
}
```
上述代码实现了 RTSP 视频流的解码,并可以通过处理解码后的图像数据实现各种功能。需要注意的是,该代码仅为示例代码,实际使用时需要根据具体情况进行修改和优化。
阅读全文
相关推荐
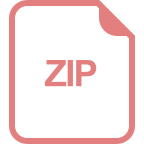

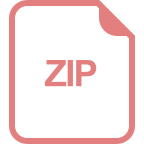
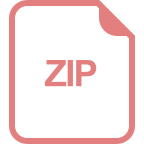
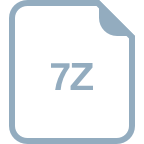
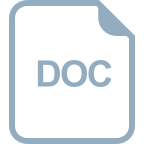
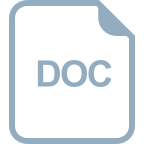







