unity json多层嵌套解析
时间: 2023-06-29 15:06:20 浏览: 226
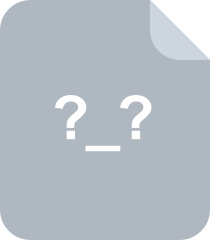
Android使用Kotlin利用Gson解析多层嵌套Json数据
在Unity中,可以使用JsonUtility类来解析Json格式的数据。对于多层嵌套的Json数据,我们可以使用JsonUtility.FromJson方法将其转换为对应的C#对象,然后再通过对象的属性或方法来访问其中的数据。
下面是一个示例Json数据:
```
{
"name": "John",
"age": 30,
"address": {
"city": "New York",
"state": "NY",
"zipcode": "10001"
},
"phoneNumbers": [
{
"type": "home",
"number": "555-1234"
},
{
"type": "work",
"number": "555-5678"
}
]
}
```
我们可以定义一个对应的C#类来表示这个Json数据:
```
[Serializable]
public class Person
{
public string name;
public int age;
public Address address;
public List<PhoneNumber> phoneNumbers;
}
[Serializable]
public class Address
{
public string city;
public string state;
public string zipcode;
}
[Serializable]
public class PhoneNumber
{
public string type;
public string number;
}
```
然后使用JsonUtility.FromJson方法将Json数据转换为Person对象:
```
string jsonData = "{...}"; // 假设这里是示例Json数据
Person person = JsonUtility.FromJson<Person>(jsonData);
```
现在我们就可以通过person对象的属性或方法来访问其中的数据了,例如:
```
Debug.Log(person.name); // 输出 "John"
Debug.Log(person.address.city); // 输出 "New York"
Debug.Log(person.phoneNumbers[0].number); // 输出 "555-1234"
```
需要注意的是,在使用JsonUtility类时,对象的属性或字段必须是public修饰的,并且类必须使用[Serializable]标记进行标记。此外,Json数据中的键名必须与C#类中的属性或字段名称相对应。如果Json数据中的键名与属性或字段名称不匹配,可以使用[JsonProperty]标记来指定对应关系。
阅读全文
相关推荐
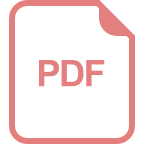
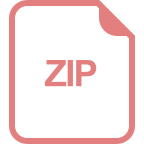
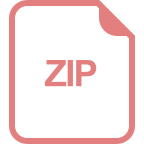
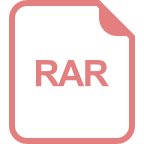
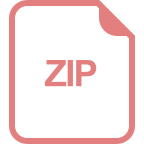
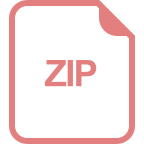
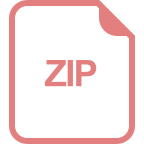
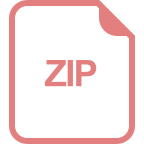
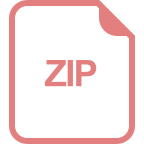
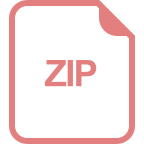
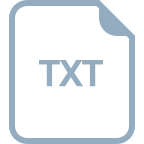