def train_loop(): step = 0 ax = [] ay = [] plt.ion() PASS_NUM = n_epochs for pass_id in range(PASS_NUM): total_loss_pass = 0 for data in train_reader(): avg_loss_value, = exe.run( fluid.default_main_program(), feed= feeder.feed(data), fetch_list=[avg_loss]) total_loss_pass += avg_loss_value ax.append(pass_id) ay.append(total_loss_pass) plt.clf() plt.plot(ax, ay) plt.pause(0.01) plt.ioff() step += 1 display.clear_output(wait=True) fluid.io.save_inference_model(SAVE_DIRNAME, ['x'], [prediction], exe) train_loop()
时间: 2024-02-14 16:35:19 浏览: 21
这段代码是一个使用 PaddlePaddle 框架进行训练的循环。具体来说,它包含以下步骤:
1. 定义了一个名为 `train_loop` 的函数,表示训练循环。
2. 初始化一些变量,包括 `step` 记录当前训练步数,`ax` 和 `ay` 用于绘制训练过程中的损失曲线,`plt.ion()` 用于打开 pyplot 的交互模式。
3. 循环执行 `PASS_NUM` 次训练,其中 `PASS_NUM` 是训练轮数。在每一轮训练中,遍历训练数据集 `train_reader()` 中的每个样本,计算平均损失值 `avg_loss_value`,并累加到 `total_loss_pass` 中。
4. 将当前轮数 `pass_id` 和累加的平均损失值 `total_loss_pass` 分别添加到 `ax` 和 `ay` 中,用于绘制损失曲线。
5. 使用 `plt.clf()` 清空之前的图像,再使用 `plt.plot(ax, ay)` 绘制当前的损失曲线,使用 `plt.pause(0.01)` 暂停一段时间,使图像得以显示。
6. 使用 `plt.ioff()` 关闭交互模式,`step` 加 1,使用 `display.clear_output(wait=True)` 清空输出界面,进入下一轮训练。
7. 训练结束后,使用 `fluid.io.save_inference_model()` 将训练好的模型保存到指定路径 `SAVE_DIRNAME` 中。
相关问题
绘制训练集和测试集的真实值和预测值图像 train_predict_plot = np.empty_like(data_scaled) train_predict_plot[:, :] = np.nan train_predict_plot[time_steps:len(train_predict) + time_steps, :] = train_predict test_predict_plot = np.empty_like(data_scaled) test_predict_plot[:, :] = np.nan test_predict_plot[len(train_predict) + time_steps * 2 + 1:len(data_scaled) - 1, :] = test_predict plt.figure(figsize=(10, 6)) plt.plot(scaler.inverse_transform(data_scaled)) plt.plot(train_predict_plot) plt.plot(test_predict_plot) plt.legend(['True', 'Train Predict', 'Test Predict']) plt.xlabel('Time/h') plt.ylabel('kwh') plt.show(改写以上代码,使得训练集部分和测试集部分分别绘制在两张图上
下面是改写后的代码,将训练集和测试集的真实值和预测值分别绘制在两张图上:
```
train_predict_plot = np.empty_like(data_scaled)
train_predict_plot[:, :] = np.nan
train_predict_plot[time_steps:len(train_predict) + time_steps, :] = train_predict
test_predict_plot = np.empty_like(data_scaled)
test_predict_plot[:, :] = np.nan
test_predict_plot[len(train_predict) + time_steps * 2 + 1:len(data_scaled) - 1, :] = test_predict
# 绘制训练集部分真实值和预测值图像
plt.figure(figsize=(10, 6))
plt.plot(scaler.inverse_transform(data_scaled[:len(train_predict)]))
plt.plot(train_predict_plot[:len(train_predict)])
plt.legend(['True', 'Train Predict'])
plt.xlabel('Time/h')
plt.ylabel('kwh')
plt.title('Training Set')
plt.show()
# 绘制测试集部分真实值和预测值图像
plt.figure(figsize=(10, 6))
plt.plot(scaler.inverse_transform(data_scaled[len(train_predict) + time_steps * 2 + 1:]))
plt.plot(test_predict_plot[len(train_predict) + time_steps * 2 + 1:])
plt.legend(['True', 'Test Predict'])
plt.xlabel('Time/h')
plt.ylabel('kwh')
plt.title('Test Set')
plt.show()
```
class RandomWalk(): def __init__(self,point_num=5000): self.point_num = point_num self.xval = [0] self.yval = [0] def fill_walk(self): while len(self.xval)<self.point_num: x_direction = choice([1,-1]) step_num = choice([0,1,2,3,4]) xstep = x_direction * step_num y_direction = choice([1,-1]) step_num = choice([0,1,2,3,4]) ystep = y_direction * step_num if xstep == 0 and ystep==0: continue x_next = self.xval[-1] + xstep y_next = self.yval[-1] + ystep self.xval.append(x_next) self.yval.append(y_next) rw = RandomWalk(50000) rw.fill_walk() point_numbers = list(range(rw.point_num())) plt.scatter(rw.x_val,rw.y_val,c=point_numbers,cmap=plt.cm.Blues,edgecolor = 'none',s=2) plt.scatter(0,0,c='green',edgecolors='none',s=100) plt.scatter(rw.x_val[-1],rw.y_val[-1],c='red',edgecolor='none',s=100) plt.axes().get_xaxis().set_visible(False) plt.axes().get_xaxis().set_yisible(False) plt.show()修改代码
以下是修改后的代码:
```python
import matplotlib.pyplot as plt
from random import choice
class RandomWalk():
def __init__(self, point_num=5000):
self.point_num = point_num
self.x_val = [0]
self.y_val = [0]
def fill_walk(self):
while len(self.x_val) < self.point_num:
x_direction = choice([1, -1])
x_step = choice([0, 1, 2, 3, 4])
x_val = x_direction * x_step
y_direction = choice([1, -1])
y_step = choice([0, 1, 2, 3, 4])
y_val = y_direction * y_step
if x_val == 0 and y_val == 0:
continue
next_x = self.x_val[-1] + x_val
next_y = self.y_val[-1] + y_val
self.x_val.append(next_x)
self.y_val.append(next_y)
rw = RandomWalk(50000)
rw.fill_walk()
point_numbers = list(range(rw.point_num))
plt.scatter(rw.x_val, rw.y_val, c=point_numbers, cmap=plt.cm.Blues, edgecolor='none', s=2)
plt.scatter(0, 0, c='green', edgecolors='none', s=100)
plt.scatter(rw.x_val[-1], rw.y_val[-1], c='red', edgecolor='none', s=100)
plt.axes().get_xaxis().set_visible(False)
plt.axes().get_yaxis().set_visible(False)
plt.show()
```
主要的修改包括:
1. `xval` 和 `yval` 改为 `x_val` 和 `y_val`,以符合 Python 的命名规范。
2. `rw.point_num()` 改为 `rw.point_num`,因为 `point_num` 是一个属性而不是方法。
3. `plt.axes().get_xaxis().set_yisible(False)` 改为 `plt.axes().get_yaxis().set_visible(False)`,因为原来的代码中打错了单词。
相关推荐
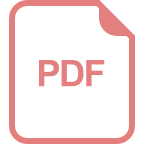












