试C语言编写程序,模拟上述管理过程。要求以顺序栈模拟停车场,以链队列模拟便道。
时间: 2023-06-01 07:02:16 浏览: 84
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10 //停车场最大容量
typedef struct {
int data[MAX_SIZE];
int top; //栈顶指针
} Stack;
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct {
Node *front; //队头指针
Node *rear; //队尾指针
} Queue;
//初始化栈
void initStack(Stack *s) {
s->top = -1;
}
//判断栈是否为空
int isStackEmpty(Stack *s) {
return s->top == -1;
}
//判断栈是否已满
int isStackFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
//进栈
void push(Stack *s, int x) {
if (isStackFull(s)) {
printf("The parking lot is full.\n");
return;
}
s->data[++s->top] = x;
printf("Car %d has parked in the parking lot.\n", x);
}
//出栈
int pop(Stack *s) {
if (isStackEmpty(s)) {
printf("The parking lot is empty.\n");
return -1;
}
int x = s->data[s->top--];
printf("Car %d has left the parking lot.\n", x);
return x;
}
//初始化队列
void initQueue(Queue *q) {
q->front = q->rear = NULL;
}
//判断队列是否为空
int isQueueEmpty(Queue *q) {
return q->front == NULL;
}
//入队
void enqueue(Queue *q, int x) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = x;
newNode->next = NULL;
if (isQueueEmpty(q)) {
q->front = q->rear = newNode;
} else {
q->rear->next = newNode;
q->rear = newNode;
}
printf("Car %d has parked in the temporary parking lot.\n", x);
}
//出队
int dequeue(Queue *q) {
if (isQueueEmpty(q)) {
printf("The temporary parking lot is empty.\n");
return -1;
}
Node *p = q->front;
int x = p->data;
q->front = p->next;
if (q->front == NULL) {
q->rear = NULL;
}
free(p);
printf("Car %d has left the temporary parking lot.\n", x);
return x;
}
int main() {
Stack s;
Queue q;
initStack(&s);
initQueue(&q);
int choice, carNum;
while (1) {
printf("Please select the operation:\n");
printf("1. Park a car\n");
printf("2. Remove a car\n");
printf("3. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Please enter the car number: ");
scanf("%d", &carNum);
push(&s, carNum);
break;
case 2:
printf("Please enter the car number: ");
scanf("%d", &carNum);
while (!isStackEmpty(&s)) {
int x = pop(&s);
if (x == carNum) {
break;
} else {
enqueue(&q, x);
}
}
while (!isQueueEmpty(&q)) {
int x = dequeue(&q);
push(&s, x);
}
break;
case 3:
exit(0);
default:
printf("Invalid choice.\n");
}
}
return 0;
}
阅读全文
相关推荐
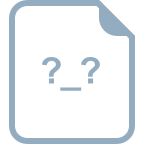
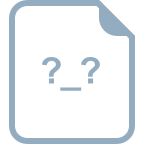
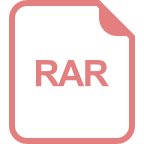
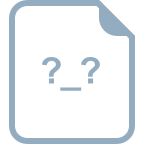
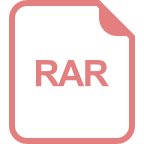
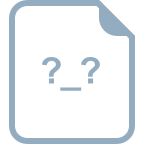
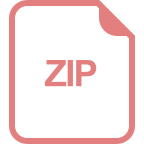
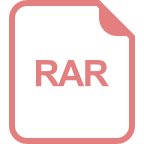
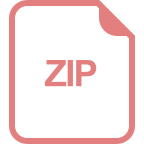
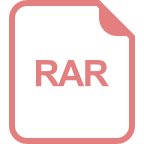