vue中倒计时应该放在哪里
时间: 2023-11-12 11:38:00 浏览: 59
Vue中的倒计时可以放在组件中的data属性中,倒计时的时间可以作为一个状态存储在data属性中。然后,在组件的生命周期钩子函数中,可以使用setInterval函数来更新倒计时的时间状态,并在模板中渲染出来。另外,也可以在Vue的计算属性中处理倒计时的逻辑,这样可以更加方便地控制倒计时的显示和隐藏。具体实现可以参考Vue的官方文档或者相关的教程。
相关问题
vue element-ui 写一个亚运会倒计时
好的,下面是一个简单的倒计时示例:
1. 安装 Element UI
首先需要安装 Element UI,可以使用以下命令:
```
npm install element-ui -S
```
2. 创建倒计时组件
在 Vue 组件中,我们可以使用 `setInterval` 函数来实现倒计时,然后将倒计时的结果渲染到页面上。下面是一个简单的倒计时组件:
```vue
<template>
<div class="countdown">
<el-row>
<el-col :span="6">
<div class="countdown-item">
<div class="countdown-value">{{ days }}</div>
<div class="countdown-label">Days</div>
</div>
</el-col>
<el-col :span="6">
<div class="countdown-item">
<div class="countdown-value">{{ hours }}</div>
<div class="countdown-label">Hours</div>
</div>
</el-col>
<el-col :span="6">
<div class="countdown-item">
<div class="countdown-value">{{ minutes }}</div>
<div class="countdown-label">Minutes</div>
</div>
</el-col>
<el-col :span="6">
<div class="countdown-item">
<div class="countdown-value">{{ seconds }}</div>
<div class="countdown-label">Seconds</div>
</div>
</el-col>
</el-row>
</div>
</template>
<script>
export default {
data() {
return {
countdownDate: new Date("2022-09-10T00:00:00.000Z")
};
},
computed: {
days() {
return Math.floor(this.remainingTime / (1000 * 60 * 60 * 24));
},
hours() {
return Math.floor(
(this.remainingTime % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)
);
},
minutes() {
return Math.floor((this.remainingTime % (1000 * 60 * 60)) / (1000 * 60));
},
seconds() {
return Math.floor((this.remainingTime % (1000 * 60)) / 1000);
},
remainingTime() {
return this.countdownDate - new Date();
}
},
mounted() {
setInterval(() => {
this.$forceUpdate();
}, 1000);
}
};
</script>
<style scoped>
.countdown {
text-align: center;
font-size: 24px;
margin-top: 50px;
}
.countdown-item {
margin-bottom: 20px;
}
.countdown-value {
font-size: 48px;
color: #409eff;
font-weight: bold;
}
.countdown-label {
font-size: 18px;
color: #666;
}
</style>
```
在这个组件中,我们定义了一个 `countdownDate` 变量来表示倒计时的结束时间。在 `computed` 中,我们计算了剩余时间,然后根据剩余时间计算天数、小时数、分钟数和秒数。最后,我们使用 `setInterval` 函数每秒钟更新一次组件。
3. 在页面中使用组件
最后,我们可以在页面中使用这个组件:
```vue
<template>
<div class="countdown-page">
<countdown />
</div>
</template>
<script>
import Countdown from "@/components/Countdown.vue";
export default {
components: {
Countdown
}
};
</script>
<style>
.countdown-page {
padding: 20px;
}
</style>
```
这个页面只是一个简单的容器,将倒计时组件放在其中。运行应用程序后,你将会看到一个简单的倒计时器。
使用app.vue写一个代码,令网页显示一个10秒的倒计时,然后显示“终于等到你!!!”
在Vue.js中,你可以使用JavaScript的`setInterval`函数来创建一个简单的10秒倒计时,然后在倒计时结束时更新页面内容。这里是一个基本的示例,我们将它放在`App.vue`组件里:
```html
<template>
<div id="app">
<h1 v-if="countdown">{{ countdown }}秒后</h1>
<p v-else>终于等到你!!!</p>
</div>
</template>
<script>
export default {
data() {
return {
countdown: null,
};
},
mounted() {
this.startCountdown();
},
methods: {
startCountdown() {
this.countdown = 10;
const intervalId = setInterval(() => {
if (this.countdown > 0) {
this.countdown--;
} else {
clearInterval(intervalId);
this.countdown = null;
}
}, 1000); // 每秒执行一次
},
},
};
</script>
```
在这个例子中,我们在`mounted`生命周期钩子启动倒计时,并在`startCountdown`方法中设置初始值为10。每次`setInterval`回调时,我们会递减`countdown`值,当倒计时到0时,清除定时器并显示"终于等到你!!!"。
阅读全文
相关推荐
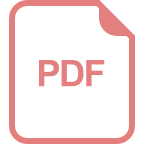
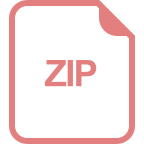
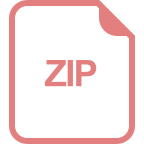
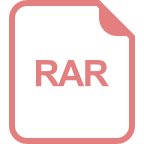
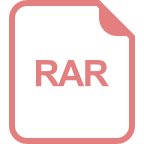
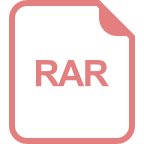
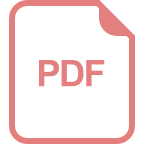
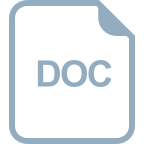
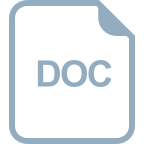

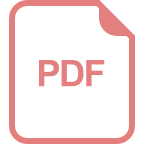
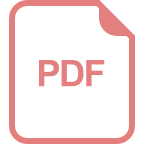
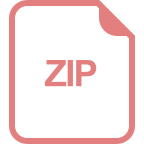
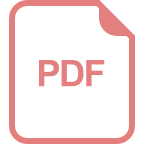
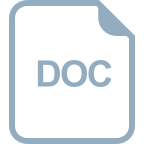
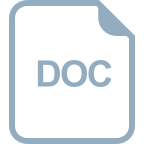
