帮我用c语言写一个图书馆管理系统
时间: 2023-11-25 18:07:14 浏览: 37
好的,下面是一个简单的图书馆管理系统的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
int id;
char title[50];
char author[50];
int available;
};
struct Library {
struct Book books[MAX_BOOKS];
int num_books;
};
void add_book(struct Library* library);
void remove_book(struct Library* library);
void search_book(struct Library* library);
void display_books(struct Library* library);
int main() {
struct Library library;
library.num_books = 0;
int choice;
do {
printf("1. Add Book\n");
printf("2. Remove Book\n");
printf("3. Search Book\n");
printf("4. Display Books\n");
printf("5. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book(&library);
break;
case 2:
remove_book(&library);
break;
case 3:
search_book(&library);
break;
case 4:
display_books(&library);
break;
case 5:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice. Try again.\n");
}
} while (choice != 5);
return 0;
}
void add_book(struct Library* library) {
if (library->num_books >= MAX_BOOKS) {
printf("Error: Library is full.\n");
return;
}
struct Book book;
book.id = library->num_books + 1;
printf("Enter the book title: ");
scanf("%s", book.title);
printf("Enter the book author: ");
scanf("%s", book.author);
book.available = 1;
library->books[library->num_books] = book;
library->num_books++;
printf("Book added successfully.\n");
}
void remove_book(struct Library* library) {
int id;
printf("Enter the book ID to remove: ");
scanf("%d", &id);
for (int i = 0; i < library->num_books; i++) {
if (library->books[i].id == id) {
for (int j = i; j < library->num_books - 1; j++) {
library->books[j] = library->books[j + 1];
}
library->num_books--;
printf("Book removed successfully.\n");
return;
}
}
printf("Error: Book not found.\n");
}
void search_book(struct Library* library) {
char keyword[50];
printf("Enter the search keyword: ");
scanf("%s", keyword);
for (int i = 0; i < library->num_books; i++) {
if (strstr(library->books[i].title, keyword) || strstr(library->books[i].author, keyword)) {
printf("%d. %s by %s", library->books[i].id, library->books[i].title, library->books[i].author);
if (library->books[i].available) {
printf(" (Available)\n");
} else {
printf(" (Not Available)\n");
}
}
}
}
void display_books(struct Library* library) {
printf("ID Title Author\n");
printf("--------------------------------------------------\n");
for (int i = 0; i < library->num_books; i++) {
printf("%-3d %-40s %-20s", library->books[i].id, library->books[i].title, library->books[i].author);
if (library->books[i].available) {
printf(" (Available)\n");
} else {
printf(" (Not Available)\n");
}
}
}
```
这个示例代码实现了以下功能:
1. 添加图书
2. 删除图书
3. 查找图书
4. 显示所有图书
5. 退出系统
您可以根据您的具体需求修改它,并添加其他功能。
相关推荐
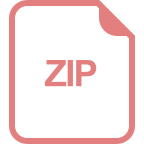
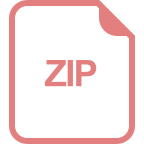
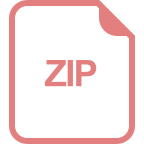
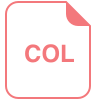
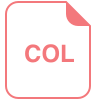
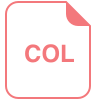
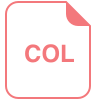
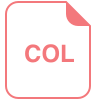









