通过srcpad获取的数据流如何获取流中profile信息
时间: 2023-10-26 18:04:46 浏览: 116
如果你想在自定义的GStreamer插件内部通过srcpad获取数据流,并获取其中的H.264 profile信息,可以使用GStreamer提供的h264parse元素。具体步骤如下:
1. 在插件的类结构体中定义一个h264parse成员变量:
```
typedef struct _MyPlugin MyPlugin;
struct _MyPlugin {
GstElement parent;
GstPad *srcpad;
GstElement *h264parse;
// other member variables
};
```
2. 在插件的类初始化函数中创建h264parse元素,并将其添加到插件的pipeline中:
```
static gboolean
my_plugin_init (GstPlugin * plugin)
{
// ...
gst_element_class_add_pad_template (klass, gst_static_pad_template_get (&src_template));
gst_element_class_add_pad_template (klass, gst_static_pad_template_get (&sink_template));
// ...
}
static void
my_plugin_class_init (MyPluginClass * klass)
{
// ...
klass->element_class->set_static_metadata (klass->element_class,
"MyPlugin",
"Generic",
"A generic plugin",
"My Name <myname@example.com>");
// ...
}
static void
my_plugin_init (MyPlugin * plugin)
{
// ...
plugin->srcpad = gst_pad_new_from_static_template (&src_template, "src");
gst_element_add_pad (GST_ELEMENT (plugin), plugin->srcpad);
plugin->h264parse = gst_element_factory_make ("h264parse", NULL);
gst_bin_add (GST_BIN (plugin), plugin->h264parse);
gst_element_link (plugin->srcpad, plugin->h264parse);
// ...
}
```
3. 在插件的srcpad的回调函数中获取数据流,并将其传递给h264parse元素:
```
static GstFlowReturn
my_plugin_srcpad_chain (GstPad * pad, GstObject * parent, GstBuffer * buf)
{
MyPlugin *plugin = MY_PLUGIN (parent);
GstFlowReturn ret = gst_pad_push (plugin->srcpad, buf);
if (ret != GST_FLOW_OK) {
return ret;
}
return GST_FLOW_OK;
}
static void
my_plugin_init (MyPlugin * plugin)
{
// ...
plugin->srcpad = gst_pad_new_from_static_template (&src_template, "src");
gst_element_add_pad (GST_ELEMENT (plugin), plugin->srcpad);
plugin->h264parse = gst_element_factory_make ("h264parse", NULL);
gst_bin_add (GST_BIN (plugin), plugin->h264parse);
gst_element_link (plugin->srcpad, plugin->h264parse);
gst_pad_set_chain_function (plugin->srcpad, my_plugin_srcpad_chain);
// ...
}
```
4. 在插件的类释放函数中释放h264parse元素:
```
static void
my_plugin_class_finalize (MyPluginClass * klass)
{
// ...
gst_element_factory_unref (GST_ELEMENT_FACTORY (klass->h264parse_factory));
// ...
}
static void
my_plugin_finalize (GObject * object)
{
MyPlugin *plugin = MY_PLUGIN (object);
// ...
gst_element_set_state (plugin->h264parse, GST_STATE_NULL);
gst_bin_remove (GST_BIN (plugin), plugin->h264parse);
gst_object_unref (GST_OBJECT (plugin->h264parse));
// ...
G_OBJECT_CLASS (my_plugin_parent_class)->finalize (object);
}
```
5. 在插件的srcpad的回调函数中获取解析器输出的H.264 profile信息:
```
static GstFlowReturn
my_plugin_srcpad_chain (GstPad * pad, GstObject * parent, GstBuffer * buf)
{
MyPlugin *plugin = MY_PLUGIN (parent);
GstFlowReturn ret = gst_pad_push (plugin->srcpad, buf);
if (ret != GST_FLOW_OK) {
return ret;
}
GstEvent *event = gst_pad_pull_event (GST_PAD (plugin->h264parse), GST_EVENT_EOS);
if (event) {
const GstStructure *structure = gst_event_get_structure (event);
if (structure) {
const gchar *name = gst_structure_get_name (structure);
if (strcmp (name, "h264parse-info") == 0) {
const GValue *value = gst_structure_get_value (structure, "profile");
if (value) {
gint profile = g_value_get_enum (value);
// process profile here
}
}
}
gst_event_unref (event);
}
return GST_FLOW_OK;
}
static void
my_plugin_init (MyPlugin * plugin)
{
// ...
plugin->srcpad = gst_pad_new_from_static_template (&src_template, "src");
gst_element_add_pad (GST_ELEMENT (plugin), plugin->srcpad);
plugin->h264parse = gst_element_factory_make ("h264parse", NULL);
gst_bin_add (GST_BIN (plugin), plugin->h264parse);
gst_element_link (plugin->srcpad, plugin->h264parse);
gst_pad_set_chain_function (plugin->srcpad, my_plugin_srcpad_chain);
// ...
}
```
上面的代码中,my_plugin_srcpad_chain是srcpad的回调函数,当有数据流从srcpad进入插件时,GStreamer会调用该函数。在该函数中,首先通过gst_pad_push函数将数据流传递给h264parse元素进行解析,然后通过gst_pad_pull_event函数从解析器的srcpad中拉取EOS事件,从而获取解析器输出的H.264 profile信息。
注意,解析器的输出信息不一定是EOS事件中的"h264parse-info"结构体中的"profile"字段,具体输出信息的结构体和字段可能会因解析器版本和配置不同而有所不同。你需要根据自己的实际情况进行调整。
阅读全文
相关推荐










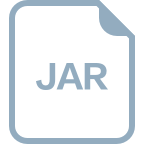




