大文件复制,基于多线程方式将大文件从目录A复制到目录B。要求通过多线程方式,线程数量可配,根据线程数与文件大小对文件进行分割,每个线程负责复制自己负责的文件块,复制到B目录整合后,文件要完成且可用?
时间: 2024-03-05 08:49:16 浏览: 52
是的,您可以使用Java的多线程技术来实现大文件复制。以下是一个基本的实现思路:
1. 首先,计算出文件的大小,然后根据线程数将文件分割成多个块。每个线程负责复制自己负责的文件块。
2. 创建一个线程池,将分割后的文件块分配给线程池中的线程去复制。
3. 每个线程复制文件块时,需要注意文件指针的位置,以确保每个线程复制的文件块不会重复或遗漏。
4. 复制完所有的文件块后,将它们整合到目标目录B中,确保文件复制完成且可用。
以下是一个简单的代码示例,用于基于多线程方式将大文件从目录A复制到目录B:
```java
import java.io.*;
public class FileCopyUtil {
private static final int THREAD_COUNT = 4; // 线程数
private static final int BUFFER_SIZE = 1024 * 1024; // 缓冲区大小
public static void copyFile(File source, File dest) throws IOException {
// 计算文件大小
long fileSize = source.length();
// 计算每个线程复制的文件块大小
long blockSize = fileSize / THREAD_COUNT;
if (fileSize % THREAD_COUNT != 0) {
blockSize++;
}
// 创建目标目录
if (!dest.exists()) {
dest.mkdirs();
}
// 创建线程池
FileCopyThread[] threads = new FileCopyThread[THREAD_COUNT];
for (int i = 0; i < THREAD_COUNT; i++) {
long start = i * blockSize;
long end = Math.min(start + blockSize, fileSize);
threads[i] = new FileCopyThread(source, dest, start, end);
}
// 启动线程池
for (FileCopyThread thread : threads) {
thread.start();
}
// 等待所有线程完成
for (FileCopyThread thread : threads) {
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 整合文件
mergeFiles(dest, threads);
}
private static void mergeFiles(File dest, FileCopyThread[] threads) throws IOException {
OutputStream out = new BufferedOutputStream(new FileOutputStream(new File(dest, threads[0].getName())));
for (FileCopyThread thread : threads) {
InputStream in = new BufferedInputStream(new FileInputStream(new File(dest, thread.getName())));
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
in.close();
}
out.close();
}
private static class FileCopyThread extends Thread {
private File source;
private File dest;
private long start;
private long end;
public FileCopyThread(File source, File dest, long start, long end) {
this.source = source;
this.dest = dest;
this.start = start;
this.end = end;
}
@Override
public void run() {
try {
BufferedInputStream in = new BufferedInputStream(new FileInputStream(source));
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(new File(dest, getName())));
byte[] buffer = new byte[BUFFER_SIZE];
int len;
in.skip(start);
while ((len = in.read(buffer)) > 0 && in.getPosition() < end) {
out.write(buffer, 0, len);
}
in.close();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
这个类将源文件和目标目录作为参数,并将文件分成多个块,然后使用线程池中的线程将块复制到目标目录。复制完成后,还将所有块整合到目标目录中。
阅读全文
相关推荐
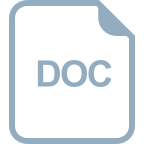
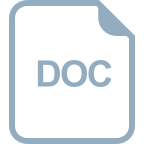
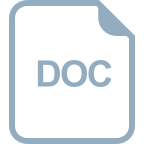
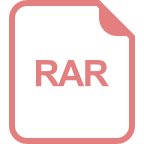
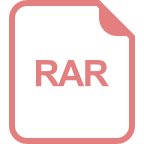
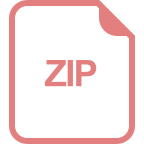
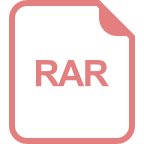
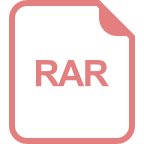
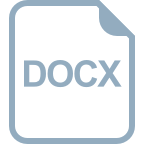
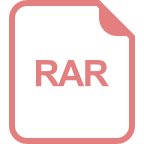
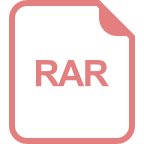
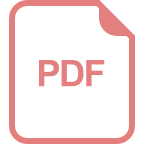
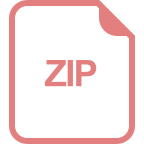
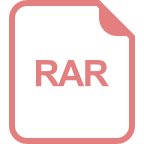
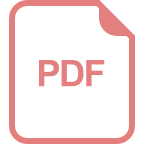
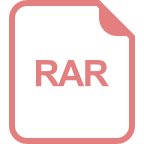