判断字符串在数组里面出现的次数
时间: 2023-11-09 13:11:29 浏览: 44
可以使用循环遍历数组,对每个元素判断是否与给定字符串相等,如果相等则计数器加1。最后返回计数器的值即可。
示例代码:
```python
def count_occurrences(arr, str):
count = 0
for item in arr:
if item == str:
count += 1
return count
```
其中,arr为待搜索的数组,str为待搜索的字符串。
相关问题
java判断字符串在数组里面出现的次数
可以采用HashMap来实现字符串在数组中出现的次数统计。
示例代码如下:
```java
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
String[] arr = {"apple", "banana", "orange", "apple", "orange", "pear", "apple"};
String targetStr = "apple";
int count = getTargetStrCount(arr, targetStr);
System.out.println(targetStr + "出现的次数为:" + count);
}
public static int getTargetStrCount(String[] arr, String targetStr) {
HashMap<String, Integer> map = new HashMap<>();
for (String str : arr) {
Integer count = map.get(str);
map.put(str, (count == null) ? 1 : count + 1);
}
return map.get(targetStr) == null ? 0 : map.get(targetStr);
}
}
```
输出结果:
```
apple出现的次数为:3
```
在此示例中,我们使用HashMap来遍历数组中的每个元素并统计其出现次数。最后,我们返回目标字符串的出现次数。
java中如何判断字符串在数组中
是否存在?
可以使用循环遍历数组,并使用equals()方法比较字符串是否相等。具体代码如下:
```java
public static boolean contains(String[] arr, String str) {
for (String s : arr) {
if (s.equals(str)) {
return true;
}
}
return false;
}
```
其中,arr是字符串数组,str是待比较的字符串。如果arr中包含str,返回true,否则返回false。
相关推荐
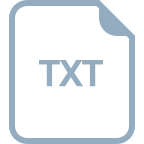
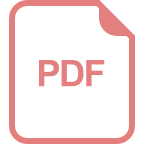
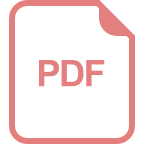












