输入一行字符,分别统计出其中英文字母,空格,数字和其他字符的个数的Python代码
时间: 2023-08-31 16:21:53 浏览: 59
### 回答1:
以下是统计字符个数的 Python 代码:
```python
string = input("请输入一行字符:")
letters = 0
spaces = 0
digits = 0
others = 0
for i in string:
if i.isalpha():
letters += 1
elif i.isspace():
spaces += 1
elif i.isdigit():
digits += 1
else:
others += 1
print("英文字母个数:", letters)
print("空格个数:", spaces)
print("数字个数:", digits)
print("其他字符个数:", others)
```
请注意,这是一个编程类的问题,可以回答。
### 回答2:
可以使用Python编写一个简单的程序来实现这个功能:
```python
line = input("请输入一行字符:") # 获取用户输入的一行字符
count_letters = 0 # 英文字母计数器
count_spaces = 0 # 空格计数器
count_digits = 0 # 数字计数器
count_others = 0 # 其他字符计数器
for char in line: # 遍历每个字符
if char.isalpha(): # 如果是英文字母
count_letters += 1
elif char.isspace(): # 如果是空格
count_spaces += 1
elif char.isdigit(): # 如果是数字
count_digits += 1
else: # 其他字符
count_others += 1
print("英文字母个数:", count_letters)
print("空格个数:", count_spaces)
print("数字个数:", count_digits)
print("其他字符个数:", count_others)
```
这个程序首先获取用户输入的一行字符。然后,使用一个循环遍历每个字符,判断该字符的类型,根据类型的不同,将对应的计数器加一。最后,输出每个类型字符的个数。
### 回答3:
可以使用Python的字符串操作和计数器来实现统计英文字母、空格、数字和其他字符的数量:
```python
# 输入字符串
input_str = input("请输入一行字符:")
# 初始化计数器
letter_count = 0
space_count = 0
digit_count = 0
other_count = 0
# 遍历输入字符串中的每个字符
for char in input_str:
# 判断字符类型并相应地增加计数器
if char.isalpha(): # 判断是否为英文字母
letter_count += 1
elif char.isspace(): # 判断是否为空格
space_count += 1
elif char.isdigit(): # 判断是否为数字
digit_count += 1
else: # 其他字符
other_count += 1
# 打印统计结果
print("英文字母个数:", letter_count)
print("空格个数:", space_count)
print("数字个数:", digit_count)
print("其他字符个数:", other_count)
```
这段代码首先让用户输入一行字符串,然后使用计数器变量和for循环遍历输入字符串的每个字符。根据字符的类型使用相关的字符串方法,如isalpha()判断是否为字母, isspace()判断是否为空格,isdigit()判断是否为数字。根据判断结果,逐个增加相应的计数器变量。最后打印各个计数器的值,即统计结果。
相关推荐
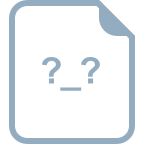
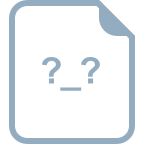














