http://www.weather.com.cn/data/sk/101010100.html将此天气API接口写入,重新生成服务器以及客户端
时间: 2023-12-19 07:03:42 浏览: 35
这是一个中国气象局的天气API接口,可以获取北京市的实时天气信息。
首先,我们需要在服务器端编写代码来获取天气数据并将其返回给客户端。以下是使用Node.js编写的示例代码:
```javascript
const http = require('http');
const API_URL = 'http://www.weather.com.cn/data/sk/101010100.html';
http.createServer((req, res) => {
if (req.url === '/weather') {
http.get(API_URL, (response) => {
let data = '';
response.on('data', (chunk) => {
data += chunk;
});
response.on('end', () => {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(data);
});
});
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not found');
}
}).listen(3000, () => {
console.log('Server running at http://localhost:3000');
});
```
该代码监听端口3000,并在访问`/weather`路径时获取天气数据并返回JSON格式的数据。
接下来,我们需要编写客户端代码来获取服务器返回的数据并显示在页面上。以下是使用jQuery编写的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>天气</title>
</head>
<body>
<div id="weather"></div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(() => {
$.getJSON('/weather', (data) => {
const weather = data.weatherinfo;
const html = `
<h1>${weather.city}</h1>
<p>温度:${weather.temp}℃</p>
<p>湿度:${weather.SD}</p>
<p>风向:${weather.WD}</p>
<p>风力:${weather.WS}</p>
`;
$('#weather').html(html);
});
});
</script>
</body>
</html>
```
该代码使用jQuery的`$.getJSON()`方法获取服务器返回的JSON格式数据,并将其显示在页面上。
最后,我们需要在命令行中启动服务器并访问页面。在命令行中执行以下命令:
```bash
node app.js
```
然后在浏览器中访问`http://localhost:3000`即可看到天气信息的页面。
相关推荐
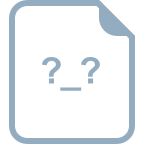
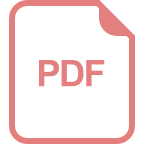
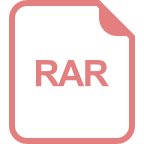














