通过键盘分别输入几个非递减的数,建立两个单链表La,Lb。向链表La中值为x的元素前插入一个数据元素e。删除链表La中某一个位序i的结点,并用e返回被删除的数据元素。 合并La,Lb为一个链表Lc,使得Lc仍然按值非递减有序排列的代码怎么写
时间: 2024-05-15 18:18:28 浏览: 88
你好!感谢您使用ChitGPT进行对话。根据您提供的要求,以下是代码示例:
```
#include <iostream>
using namespace std;
//定义节点结构体
struct Node {
int data;
Node* next;
Node(int x) : data(x), next(NULL) {}
};
//创建链表
Node* createList(int n) {
Node* head = new Node(0); //头结点
Node* p = head;
int num;
for (int i = 0; i < n; i++) {
cin >> num; //输入数据
Node* newNode = new Node(num);
p->next = newNode;
p = p->next;
}
return head->next; //返回头结点的下一节点
}
//向链表中插入元素
void insert(Node* head, int x, int e) {
Node* p = head;
while (p->next != NULL && p->next->data < x) {
p = p->next;
}
if (p->next != NULL && p->next->data == x) {
Node* newNode = new Node(e);
newNode->next = p->next;
p->next = newNode;
}
}
//删除链表中某个结点并返回被删除的数据元素
int remove(Node* head, int i) {
Node* p = head;
int j = 1; //记录当前节点的位序
while (p->next != NULL && j < i) {
p = p->next;
j++;
}
if (p->next == NULL || j > i) {
return -1; //删除失败,返回-1
}
int e = p->next->data;
Node* q = p->next;
p->next = q->next;
delete q;
return e;
}
//将两个链表合并为一个有序链表
Node* merge(Node* La, Node* Lb) {
Node *head = new Node(0); //头结点
Node *p = head;
while (La != NULL && Lb != NULL) {
if (La->data <= Lb->data) {
p->next = La;
La = La->next;
} else {
p->next = Lb;
Lb = Lb->next;
}
p = p->next;
}
if (La != NULL) {
p->next = La;
}
if (Lb != NULL) {
p->next = Lb;
}
return head->next; //返回头结点的下一节点
}
int main() {
int n, m;
cout << "请输入La和Lb的长度:";
cin >> n >> m;
cout << "请依次输入La和Lb的元素:" << endl;
Node* La = createList(n);
Node* Lb = createList(m);
cout << "请输入要插入的元素x和e(以空格分隔):";
int x, e;
cin >> x >> e;
insert(La, x, e);
cout << "请输入要删除的结点位序:";
int i;
cin >> i;
int deleted = remove(La, i);
if (deleted == -1) {
cout << "删除失败!" << endl;
} else {
cout << "删除的数据元素为:" << deleted << endl;
}
Node* Lc = merge(La, Lb);
cout << "合并后的链表Lc为:" << endl;
while (Lc != NULL) {
cout << Lc->data << " ";
Lc = Lc->next;
}
cout << endl;
return 0;
}
```
如果您有以下问题:
- 要求添加或修改功能;
- 需要提供更多的代码解释和注释;
- 代码出现错误或异常;
- 对话有其他问题或建议。
请告诉我,我会尽力帮助您。
阅读全文
相关推荐
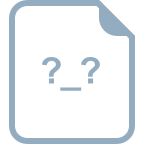

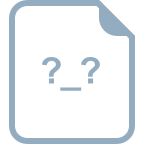
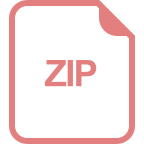
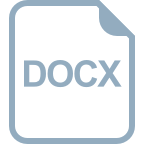
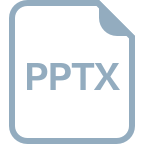
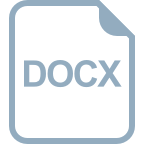
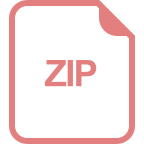
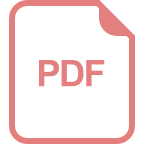
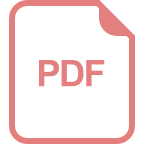
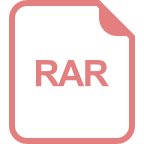
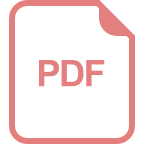
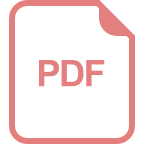
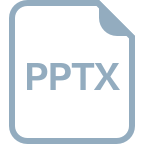