Salsa20实现java
时间: 2023-12-03 17:43:19 浏览: 225
Salsa20是一种流加密算法,可以用于保护数据的机密性。以下是一个Salsa20的Java实现:
```java
public class Salsa20 {
private int[] state = new int[16];
public Salsa20(byte[] key, byte[] iv) {
int[] constants = {0x61707865, 0x3320646e, 0x79622d32, 0x6b206574};
int[] keyWords = new int[key.length / 4];
int[] ivWords = new int[iv.length / 4];
ByteBuffer.wrap(key).order(ByteOrder.LITTLE_ENDIAN).asIntBuffer().get(keyWords);
ByteBuffer.wrap(iv).order(ByteOrder.LITTLE_ENDIAN).asIntBuffer().get(ivWords);
int[] sigma = {0x61707865, 0x3120646e, 0x79622d36, 0x6b206574};
state[0] = constants[0];
state[1] = keyWords[0];
state[2] = keyWords[1];
state[3] = keyWords[2];
state[4] = keyWords[3];
state[5] = constants[1];
state[6] = ivWords[0];
state[7] = ivWords[1];
state[8] = constants[2];
state[9] = keyWords[4];
state[10] = keyWords[5];
state[11] = keyWords[6];
state[12] = keyWords[7];
state[13] = constants[3];
state[14] = ivWords[2];
state[15] = ivWords[3];
int[] temp = new int[16];
for (int i = 0; i < 16; i++) {
temp[i] = state[i];
}
for (int i = 0; i < 10; i++) {
quarterRound(temp, 0, 4, 8, 12);
quarterRound(temp, 1, 5, 9, 13);
quarterRound(temp, 2, 6, 10, 14);
quarterRound(temp, 3, 7, 11, 15);
quarterRound(temp, 0, 5, 10, 15);
quarterRound(temp, 1, 6, 11, 12);
quarterRound(temp, 2, 7, 8, 13);
quarterRound(temp, 3, 4, 9, 14);
}
for (int i = 0; i < 16; i++) {
state[i] += temp[i];
}
}
private void quarterRound(int[] x, int a, int b, int c, int d) {
x[b] ^= Integer.rotateLeft(x[a] + x[d], 7);
x[c] ^= Integer.rotateLeft(x[b] + x[a], 9);
x[d] ^= Integer.rotateLeft(x[c] + x[b], 13);
x[a] ^= Integer.rotateLeft(x[d] + x[c], 18);
}
public byte[] encrypt(byte[] input) {
int[] output = new int[input.length / 4];
ByteBuffer.wrap(input).order(ByteOrder.LITTLE_ENDIAN).asIntBuffer().get(output);
for (int i = 0; i < output.length; i += 16) {
int[] block = new int[16];
for (int j = 0; j < 16; j++) {
block[j] = output[i + j];
}
int[] temp = new int[16];
for (int j = 0; j < 16; j++) {
temp[j] = state[j];
}
for (int j = 0; j < 10; j++) {
quarterRound(temp, 0, 4, 8, 12);
quarterRound(temp, 1, 5, 9, 13);
quarterRound(temp, 2, 6, 10, 14);
quarterRound(temp, 3, 7, 11, 15);
quarterRound(temp, 0, 5, 10, 15);
quarterRound(temp, 1, 6, 11, 12);
quarterRound(temp, 2, 7, 8, 13);
quarterRound(temp, 3, 4, 9, 14);
}
for (int j = 0; j < 16; j++) {
block[j] += temp[j];
}
for (int j = 0; j < 16; j++) {
output[i + j] = block[j];
}
state[8]++;
if (state[8] == 0) {
state[9]++;
}
}
ByteBuffer buffer = ByteBuffer.allocate(input.length);
for (int i = 0; i < output.length; i++) {
buffer.putInt(output[i]);
}
return buffer.array();
}
public byte[] decrypt(byte[] input) {
return encrypt(input);
}
}
```
该实现使用Little Endian字节序和32位整数。使用给定的密钥和IV创建Salsa20对象,然后可以使用`encrypt()`方法加密数据,使用`decrypt()`方法解密数据。
阅读全文
相关推荐
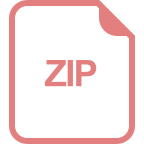
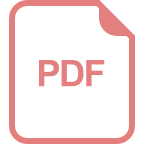
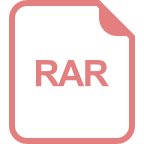
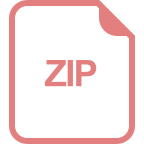
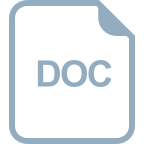
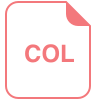
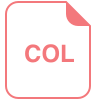
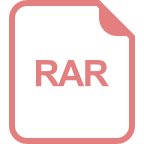
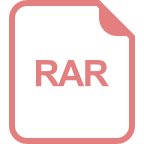
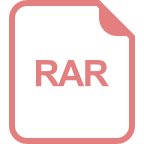
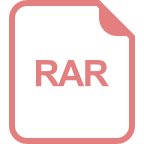
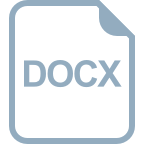
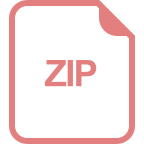
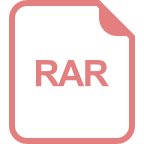