商品订购系统使用c++编程
时间: 2023-08-06 12:05:57 浏览: 143
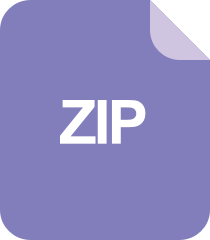
C++实现的简单购物系统

好的,下面是一个简单的商品订购系统的C++代码示例,您可以参考它来编写自己的程序:
```
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 商品类
class Product {
public:
string name; // 商品名称
double price; // 商品价格
Product(string name, double price) {
this->name = name;
this->price = price;
}
};
// 订单类
class Order {
public:
vector<Product> products; // 商品列表
// 添加商品到订单中
void addProduct(Product product) {
products.push_back(product);
}
// 获取订单总金额
double getTotalPrice() {
double totalPrice = 0;
for (Product product : products) {
totalPrice += product.price;
}
return totalPrice;
}
};
int main() {
// 创建商品
Product p1("苹果", 5.0);
Product p2("香蕉", 3.0);
Product p3("橙子", 4.0);
// 创建订单
Order order;
order.addProduct(p1);
order.addProduct(p2);
order.addProduct(p3);
// 输出订单信息
cout << "订单详情:" << endl;
for (Product product : order.products) {
cout << product.name << " ¥" << product.price << endl;
}
cout << "总金额:¥" << order.getTotalPrice() << endl;
return 0;
}
```
这个程序中定义了两个类:`Product`和`Order`,前者表示商品,后者表示订单。在`main`函数中,我们创建了三个商品,并将它们添加到了一个订单中。最后,我们输出了订单的详细信息和总金额。您可以根据自己的需求来修改和扩展这个程序。
阅读全文
相关推荐
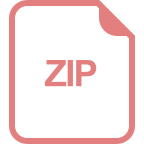
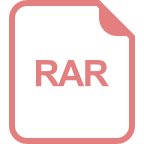
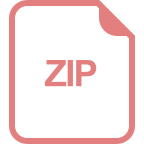
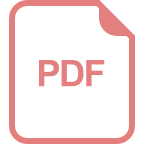
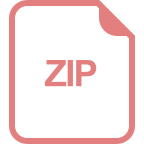
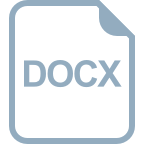
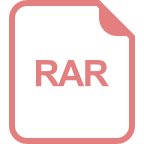
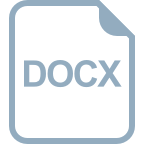
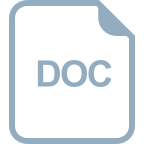
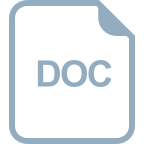
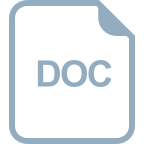
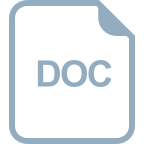
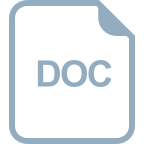
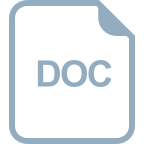