通过HTML+CSS实现管理员登录页面布局 为管理员账号和密码输入框添加placeholder与required属性 通过jQuery选择页面元素,实现管理员登录 当管理员账号为“admin”,密码为“123456”时,则登录成功 当账户与密码错误时,弹出“用户名或密码不正确,请重新填写!”提示对话框
时间: 2024-03-12 21:45:04 浏览: 139
好的,根据您的要求,我可以给出一个简单的示例代码,实现管理员登录页面布局、输入框属性设置、jQuery元素选择和登录验证等功能。
HTML代码:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>管理员登录</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="login-box">
<h2>管理员登录</h2>
<form>
<div class="user-box">
<input type="text" name="username" placeholder="请输入管理员账号" required>
</div>
<div class="user-box">
<input type="password" name="password" placeholder="请输入管理员密码" required>
</div>
<button type="submit" class="submit-btn">登录</button>
</form>
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="script.js"></script>
</body>
</html>
```
CSS代码:
```css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
background: #f7f7f7;
}
.login-box {
width: 400px;
height: 430px;
background: #fff;
color: #333;
top: 50%;
left: 50%;
position: absolute;
transform: translate(-50%,-50%);
box-shadow: 0 10px 20px rgba(0,0,0,.1);
padding: 70px 30px;
border-radius: 10px;
}
.login-box h2 {
font-size: 22px;
margin-bottom: 30px;
}
.user-box {
position: relative;
}
.user-box input {
width: 100%;
padding: 10px 0;
font-size: 16px;
letter-spacing: 1px;
margin-bottom: 30px;
border: none;
outline: none;
background: transparent;
border-bottom: 1px solid #333;
}
.user-box label {
position: absolute;
top: 0;
left: 0;
letter-spacing: 1px;
padding: 10px 0;
font-size: 16px;
color: #333;
pointer-events: none;
transition: .5s;
}
.user-box input:focus ~ label,
.user-box input:valid ~ label {
top: -20px;
left: 0;
color: #ff652f;
font-size: 14px;
}
.submit-btn {
background: #ff652f;
color: #fff;
border: none;
outline: none;
border-radius: 30px;
cursor: pointer;
font-size: 18px;
font-weight: bold;
padding: 10px 20px;
margin-top: 20px;
transition: .5s;
}
.submit-btn:hover {
background: #333;
}
```
JavaScript代码:
```javascript
$(function() {
// 监听登录表单提交事件
$('form').submit(function(e) {
e.preventDefault(); // 阻止表单默认提交事件
// 获取账号和密码输入框的值
var username = $('input[name="username"]').val();
var password = $('input[name="password"]').val();
// 验证账号和密码是否正确
if (username === 'admin' && password === '123456') {
alert('登录成功');
} else {
alert('用户名或密码不正确,请重新填写!');
}
});
});
```
根据以上代码,可以实现一个简单的管理员登录页面,包括输入框的占位符和必填属性设置、jQuery元素选择和登录验证等功能。当管理员输入正确的账号和密码时,会弹出“登录成功”提示框;当管理员输入错误的账号和密码时,会弹出“用户名或密码不正确,请重新填写!”提示框。
阅读全文
相关推荐
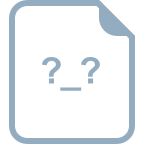
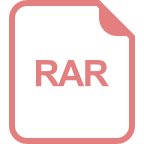
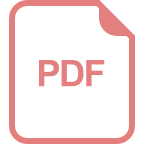
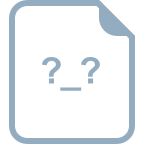
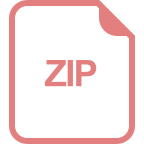

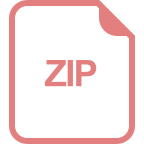
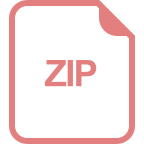
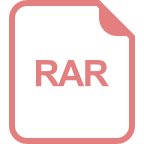
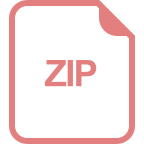
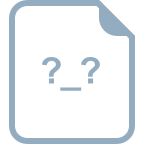
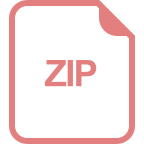
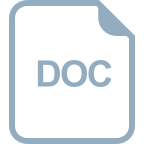
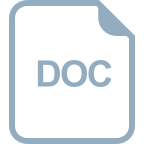
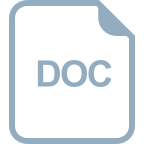
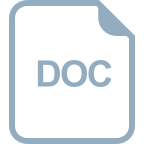


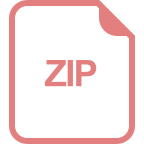