后端springboot 前端vue 导出Excel 返回JsonObject 对象 相关blob封装在jsonObject中
时间: 2024-03-12 13:49:40 浏览: 26
好的,那么您可以使用Apache POI库来实现Excel的导出,同时将生成的Excel文件的字节数组(即Blob)封装到一个JsonObject对象中,然后返回该对象给前端。
具体实现步骤如下:
1. 后端使用Apache POI库创建Excel文件并写入数据。
2. 在Controller中定义一个导出Excel的接口,使用JsonObject对象来封装Excel文件的字节数组和其他相关信息。
3. 将JsonObject对象转换成字符串后,使用ResponseEntity将其写入响应体中,并设置Content-Type为application/json,这样前端就可以通过解析响应体得到Excel文件的字节数组和其他相关信息。
4. 如果导出Excel遇到异常,可以使用try-catch语句捕获异常,并将异常信息封装到JsonObject对象中返回给前端。
下面是一个简单的示例代码,仅供参考:
```java
@RestController
public class ExcelController {
@GetMapping("/exportExcel")
public ResponseEntity<String> exportExcel() {
JsonObject result = new JsonObject();
try {
// 使用Apache POI创建Excel文件并写入数据
Workbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet("sheet1");
Row row = sheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue("Hello World!");
ByteArrayOutputStream out = new ByteArrayOutputStream();
wb.write(out);
// 将生成的Excel文件的字节数组封装到JsonObject对象中
result.addProperty("success", true);
result.addProperty("message", "导出Excel成功");
result.addProperty("fileName", "example.xls");
result.addProperty("fileContent", Base64.getEncoder().encodeToString(out.toByteArray()));
// 将JsonObject对象转换成字符串并返回给前端
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
return new ResponseEntity<>(result.toString(), headers, HttpStatus.OK);
} catch (Exception e) {
// 如果导出Excel遇到异常,将异常信息封装到JsonObject对象中返回给前端
result.addProperty("success", false);
result.addProperty("message", "导出Excel失败:" + e.getMessage());
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
return new ResponseEntity<>(result.toString(), headers, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
```
在前端中,您可以使用axios或其他HTTP库来调用后端的导出Excel接口,例如:
```javascript
axios.get('/exportExcel')
.then(response => {
if (response.data.success) {
// 导出Excel成功,使用Blob对象创建一个URL并下载Excel文件
let url = URL.createObjectURL(base64ToBlob(response.data.fileContent));
let link = document.createElement('a');
link.style.display = 'none';
link.href = url;
link.setAttribute('download', response.data.fileName);
document.body.appendChild(link);
link.click();
} else {
// 导出Excel失败,展示错误信息
console.error(response.data.message);
alert('导出Excel失败');
}
})
.catch(error => {
// 调用导出Excel接口失败,展示错误信息
console.error(error);
alert('调用导出Excel接口失败');
});
function base64ToBlob(base64String) {
let byteCharacters = atob(base64String);
let byteNumbers = new Array(byteCharacters.length);
for (let i = 0; i < byteCharacters.length; i++) {
byteNumbers[i] = byteCharacters.charCodeAt(i);
}
let byteArray = new Uint8Array(byteNumbers);
return new Blob([byteArray]);
}
```
这样就可以实现将生成的Excel文件的字节数组封装到JsonObject对象中,然后返回该对象给前端的功能了。
相关推荐
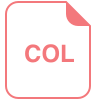
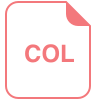
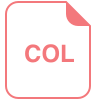
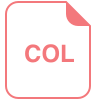










