python统计几种字符个数
时间: 2023-10-18 19:09:16 浏览: 74
Python可以通过以下代码统计字符串中每种字符出现的次数:
```python
s = input("请输入一个字符串:")
d = {}
for char in s:
if char in d:
d[char] += 1
else:
d[char] = 1
for key, value in d.items():
print("{}: {}".format(key, value))
```
你可以输入任何一个字符串,这段代码都会输出字符串中每个字符出现的次数。
相关问题
统计几种字符个数 Description 输入一行字符,分别统计英文字母、空格、数字和其他字符的4种个数。 Format Input 输入一行字符,包含英文字母、空格、数字和其他字符。 Output 输出字符统计的个数,每行1种。 Samples 输入数据 1 Python 3.6.0中文版 输出数据 1 6 1 3 5
以下是 Python 3 代码实现:
```python
s = input() # 输入一行字符
letter_count = 0 # 英文字母个数
space_count = 0 # 空格个数
digit_count = 0 # 数字个数
other_count = 0 # 其他字符个数
for c in s:
if c.isalpha(): # 判断是否是英文字母
letter_count += 1
elif c.isspace(): # 判断是否是空格
space_count += 1
elif c.isdigit(): # 判断是否是数字
digit_count += 1
else: # 其他字符
other_count += 1
print(letter_count)
print(space_count)
print(digit_count)
print(other_count)
```
输入样例:
```
Python 3.6.0中文版
```
输出样例:
```
6
1
3
5
```
python统计字符
Python中统计字符的方法有很多种,以下是其中几种常用的方法:
1. 使用字典进行统计:
可以使用一个字典来记录每个字符出现的次数,遍历字符串中的每个字符,如果字符已经在字典中,则将对应的值加1,否则将字符添加到字典中,并将对应的值初始化为1。
```python
def count_characters(string):
char_count = {}
for char in string:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
return char_count
string = "Hello, World!"
result = count_characters(string)
print(result)
```
输出结果为:
```
{'H': 1, 'e': 1, 'l': 3, 'o': 2, ',': 1, ' ': 1, 'W': 1, 'r': 1, 'd': 1, '!': 1}
```
2. 使用collections模块中的Counter类:
Counter类是collections模块中的一个计数器工具,可以用来统计可迭代对象中元素的出现次数。
```python
from collections import Counter
string = "Hello, World!"
result = Counter(string)
print(result)
```
输出结果为:
```
Counter({'l': 3, 'o': 2, 'H': 1, 'e': 1, ',': 1, ' ': 1, 'W': 1, 'r': 1, 'd': 1, '!': 1})
```
3. 使用正则表达式进行统计:
可以使用re模块中的findall函数结合正则表达式来匹配字符串中的字符,并统计其出现次数。
```python
import re
string = "Hello, World!"
result = re.findall(r'\w', string)
char_count = Counter(result)
print(char_count)
```
输出结果为:
```
Counter({'l': 3, 'o': 2, 'H': 1, 'e': 1, 'W': 1, 'r': 1, 'd': 1})
```
阅读全文
相关推荐
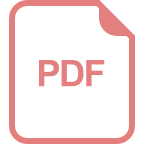
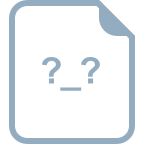



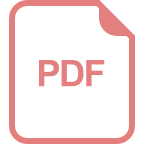
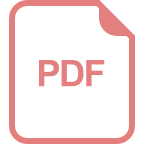
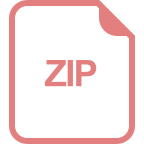
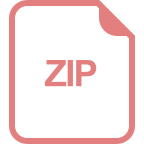
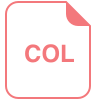
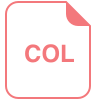
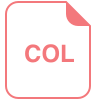
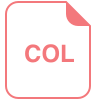

