用python画五棵樱花树和飘落的樱花
时间: 2024-05-07 11:18:43 浏览: 74
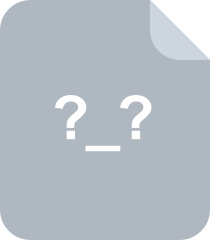
python画樱花树
好的,下面是一份使用 Python 绘制五棵樱花树和飘落的樱花的代码:
```python
import turtle
import random
# 画樱花的枝干
def draw_tree(branch_len):
if branch_len > 10:
# 随机画笔颜色
turtle.pencolor('brown')
# 设置画笔粗细
turtle.pensize(branch_len / 10)
# 向前画出枝干的长度
turtle.forward(branch_len)
# 随机角度
angle = random.uniform(15, 45)
# 向右旋转一定角度
turtle.right(angle)
# 递归画出右侧的枝干
draw_tree(branch_len - random.uniform(10, 20))
# 向左旋转一定角度
turtle.left(angle * 2)
# 递归画出左侧的枝干
draw_tree(branch_len - random.uniform(10, 20))
# 返回上一个节点
turtle.right(angle)
# 向后撤回画笔
turtle.backward(branch_len)
# 画樱花
def draw_flower(petal_num):
# 随机画笔颜色
turtle.pencolor('pink')
# 设置画笔粗细
turtle.pensize(2)
# 随机花瓣数量
petals = petal_num + random.randint(-2, 2)
# 随机花瓣大小
petal_size = random.uniform(10, 20)
# 绘制花瓣
for _ in range(petals):
turtle.forward(petal_size)
turtle.right(360 / petals)
turtle.circle(1)
turtle.right(180 - 360 / petals)
turtle.forward(petal_size)
turtle.right(180 - 360 / petals)
# 飘落的樱花
def snow(num):
# 随机画笔颜色
turtle.pencolor('white')
# 随机画笔粗细
turtle.pensize(2)
# 随机花瓣大小
petal_size = random.uniform(10, 20)
# 随机花瓣数量
petals = random.randint(4, 8)
# 绘制花瓣
for _ in range(petals):
turtle.forward(petal_size)
turtle.right(360 / petals)
turtle.circle(1)
turtle.right(180 - 360 / petals)
turtle.forward(petal_size)
turtle.right(180 - 360 / petals)
# 随机移动位置
turtle.penup()
x = random.uniform(-200, 200)
y = random.uniform(200, 400)
turtle.goto(x, y)
turtle.pendown()
# 随机飘落速度
speed = random.uniform(5, 10)
for i in range(num):
turtle.right(random.uniform(-90, 90))
turtle.forward(speed)
# 设置画布大小
turtle.setup(800, 600)
# 隐藏画笔
turtle.hideturtle()
# 加速绘制速度
turtle.tracer(100)
# 设置画笔起始位置
turtle.penup()
turtle.goto(0, -200)
turtle.pendown()
# 绘制五棵樱花树
for _ in range(5):
draw_tree(100)
turtle.penup()
x = random.uniform(-300, 300)
y = random.uniform(-200, -100)
turtle.goto(x, y)
turtle.pendown()
draw_flower(8)
# 隐藏画笔
turtle.hideturtle()
# 绘制飘落的樱花
for i in range(200):
snow(10)
# 点击关闭窗口
turtle.exitonclick()
```
运行以上代码,即可在画布上看到五棵樱花树和飘落的樱花效果。
阅读全文
相关推荐
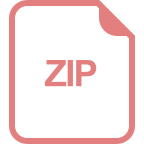
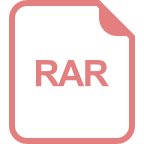
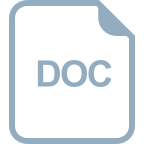

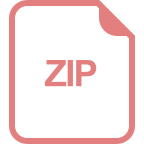
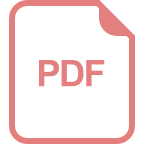
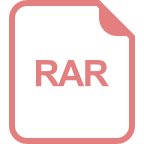
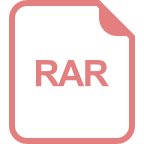
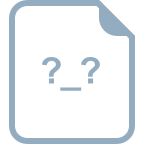
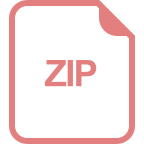
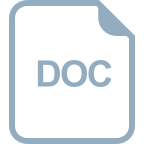
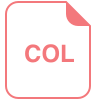
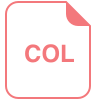
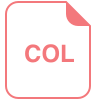

