linux 用c语言遍历
时间: 2024-05-10 18:18:27 浏览: 6
遍历什么呢?文件系统目录结构,或者说是链表、数组等数据结构?以下是一些遍历的示例代码:
1. 遍历文件夹中的所有文件和子文件夹
```c
#include <stdio.h>
#include <dirent.h>
void listdir(const char *path) {
struct dirent *entry;
DIR *dir = opendir(path);
if (dir == NULL) {
return;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_DIR) {
char path2[1024];
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
snprintf(path2, sizeof(path2), "%s/%s", path, entry->d_name);
listdir(path2);
} else {
printf("%s/%s\n", path, entry->d_name);
}
}
closedir(dir);
}
int main() {
listdir("/path/to/folder");
return 0;
}
```
2. 遍历链表
```c
#include <stdio.h>
struct node {
int data;
struct node *next;
};
void traverse_list(struct node *head) {
while (head != NULL) {
printf("%d\n", head->data);
head = head->next;
}
}
int main() {
struct node *head = NULL;
// add nodes to linked list
traverse_list(head);
return 0;
}
```
3. 遍历数组
```c
#include <stdio.h>
void traverse_array(int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d\n", arr[i]);
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
traverse_array(arr, size);
return 0;
}
```
相关推荐
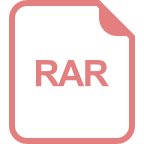
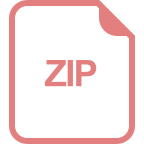














