http::response<http::string_body> res{ http::status::ok, req.version() }; res.set(http::field::server, BOOST_BEAST_VERSION_STRING); res.set(http::field::content_type, "application/json"); res.keep_alive(req.keep_alive()); res.body() = jsonData; res.prepare_payload(); http::write(socket, res);响应openCV的Mat到浏览器
时间: 2024-02-06 15:03:52 浏览: 24
如果你想要响应OpenCV的Mat数据到浏览器中,可以将Mat数据编码为JPEG或PNG格式的数据,然后将其作为响应体发送给客户端。以下是一个示例代码:
```c++
#include <iostream>
#include <boost/asio.hpp>
#include <opencv2/opencv.hpp>
#include <boost/beast.hpp>
using namespace std;
using namespace boost::asio;
int main()
{
io_service service;
ip::tcp::acceptor acceptor(service, ip::tcp::endpoint(ip::tcp::v4(), 8080));
cout << "Server started on port 8080" << endl;
while (true)
{
ip::tcp::socket socket(service);
acceptor.accept(socket);
cv::VideoCapture cap(0);
if (!cap.isOpened()) {
cerr << "Failed to open camera" << endl;
return -1;
}
cv::Mat frame;
vector<uchar> buf;
vector<int> params = {cv::IMWRITE_JPEG_QUALITY, 80};
string contentType = "image/jpeg";
while (true)
{
cap >> frame;
cv::imencode(".jpg", frame, buf, params);
string body(buf.begin(), buf.end());
boost::beast::http::response<boost::beast::http::string_body> res{ boost::beast::http::status::ok, 11 };
res.set(boost::beast::http::field::server, BOOST_BEAST_VERSION_STRING);
res.set(boost::beast::http::field::content_type, contentType);
res.keep_alive(true);
res.body() = body;
res.prepare_payload();
boost::beast::http::write(socket, res);
if (!res.keep_alive()) {
break;
}
}
}
return 0;
}
```
该示例程序会启动一个服务器,监听端口8080。当有客户端连接成功后,它会从摄像头中获取图像数据,将其编码为JPEG格式的数据,并将其作为响应体发送给客户端。浏览器可以通过访问http://localhost:8080来查看实时视频。
注意,该示例程序中的响应格式为HTTP协议的响应格式,可以根据需要进行调整。另外,该示例程序中没有考虑多线程等并发问题,仅供参考。如果需要实现更复杂的功能,可以参考boost asio和boost beast文档进行扩展。
相关推荐
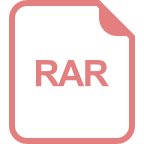














