index.stream().reduce((first, second) -> second)
时间: 2023-12-18 22:03:21 浏览: 87
This line of code uses the reduce() method to get the last element in the index stream.
The reduce() method takes a BinaryOperator as an argument, which in this case is a lambda expression (first, second) -> second. This lambda expression takes two elements from the stream and returns the second element.
As reduce() processes the stream, it applies the lambda expression to the first and second elements, then applies it again to the result and the next element, and so on until there are no more elements in the stream.
Since the lambda expression always returns the second element, reduce() will eventually return the last element in the stream.
相关问题
java stream 最后一个
在Java Stream中,可以使用Stream的reduce()方法来获取Stream中的最后一个元素。例如:
```
List<Integer> list = Arrays.asList(1, 2, 3, 4, 5);
Optional<Integer> lastElement = list.stream().reduce((first, second) -> second);
if(lastElement.isPresent()) {
System.out.println("最后一个元素是:" + lastElement.get());
} else {
System.out.println("Stream为空,没有最后一个元素");
}
```
在这个代码段中,我们使用Stream的reduce()方法,将第一个元素作为初始值,然后将第二个元素作为操作数传递给Lambda表达式。在Lambda表达式中,我们将第二个元素作为返回值,将其作为下一次操作的第一个元素。当Stream中只有一个元素时,reduce()方法将返回该元素。当Stream为空时,reduce()方法将返回一个空的Optional对象。我们可以使用isPresent()方法来检查Optional对象是否为空,如果不为空,我们可以使用get()方法来获取其值。
list stream流根据某个字段去重 取最后一个元素
可以使用Java 8中的Stream API来实现。具体步骤如下:
1. 首先,使用`distinct()`方法根据某个字段进行去重。
2. 接着,使用`sorted()`方法按照该字段进行排序,以便取最后一个元素。
3. 最后,使用`reduce()`方法将整个流缩减为一个元素,取其中的最后一个元素。
例如,假设我们有一个`List`对象,其中包含一些`Person`对象,每个`Person`对象都有一个名字和年龄属性。我们想要根据名字进行去重,并且取年龄最大的那个人,可以按照以下方式实现:
```java
List<Person> people = ... // 初始化List
Person latestPerson = people.stream()
.distinct() // 根据名字去重
.sorted(Comparator.comparing(Person::getName).thenComparing(Person::getAge).reversed()) // 按照名字和年龄进行排序
.reduce((first, second) -> second) // 取最后一个元素
.orElse(null); // 如果流为空,则返回null
System.out.println(latestPerson.getName() + " is the latest person with age " + latestPerson.getAge());
```
上面的代码定义了一个名为`latestPerson`的变量,它是通过对`people`列表进行流处理得到的。首先,用`distinct()`方法对名字进行去重;然后,使用`sorted()`方法按照名字和年龄进行排序,以便取最后一个元素;最后,使用`reduce()`方法将整个流缩减为一个元素,并取得其中的最后一个元素。如果流为空,则返回null。最后,将`latestPerson`的名字和年龄打印出来。
阅读全文
相关推荐
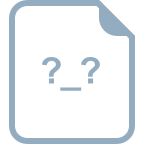
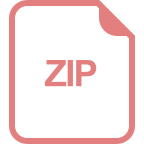
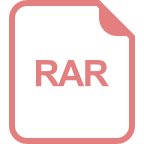
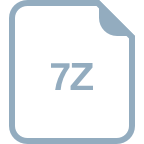
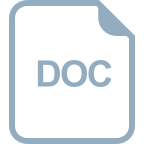
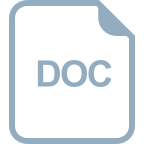
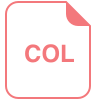
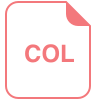
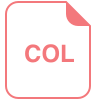
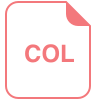
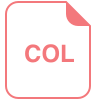
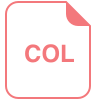
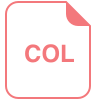
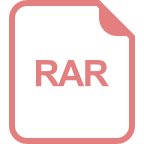
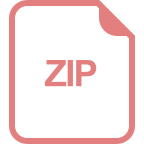