请按照自己的理解,对照《Springboot初级课件》,描述SpringBoot整合SpringMVC+MyBatis的步骤,及如何实现插入用户的功能,要求有步骤,代码,截图
时间: 2024-04-04 19:29:26 浏览: 24
整合SpringMVC和MyBatis是Spring Boot的一个常见应用场景。下面是整合SpringMVC和MyBatis的步骤:
1. 创建一个Spring Boot项目,可以使用Spring Initializr快速创建一个Maven项目。
2. 在pom.xml文件中添加Spring MVC和MyBatis的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.2</version>
</dependency>
```
3. 在src/main/resources目录下创建application.properties文件,并添加以下内容:
```properties
# 数据源配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&autoReconnect=true&failOverReadOnly=false&zeroDateTimeBehavior=convertToNull
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# MyBatis配置
mybatis.type-aliases-package=com.example.model
mybatis.mapper-locations=classpath:mapper/*.xml
```
4. 在src/main/java目录下创建一个Model类,用于映射数据库表:
```java
public class User {
private Long id;
private String name;
private Integer age;
// 省略getter和setter方法
}
```
5. 在src/main/java目录下创建一个Mapper接口,用于定义数据库操作方法:
```java
public interface UserMapper {
List<User> getAll();
User getById(Long id);
void insert(User user);
void update(User user);
void delete(Long id);
}
```
6. 在src/main/resources目录下创建一个mapper目录,并在其中创建一个userMapper.xml文件,用于编写SQL语句:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.model.User">
<id column="id" property="id" jdbcType="BIGINT" />
<result column="name" property="name" jdbcType="VARCHAR" />
<result column="age" property="age" jdbcType="INTEGER" />
</resultMap>
<select id="getAll" resultMap="BaseResultMap">
SELECT id, name, age
FROM user
</select>
<select id="getById" resultMap="BaseResultMap" parameterType="java.lang.Long">
SELECT id, name, age
FROM user
WHERE id = #{id}
</select>
<insert id="insert" parameterType="com.example.model.User">
INSERT INTO user(name, age)
VALUES(#{name}, #{age})
</insert>
<update id="update" parameterType="com.example.model.User">
UPDATE user
SET name = #{name}, age = #{age}
WHERE id = #{id}
</update>
<delete id="delete" parameterType="java.lang.Long">
DELETE FROM user
WHERE id = #{id}
</delete>
</mapper>
```
7. 在src/main/java目录下创建一个Controller类,用于处理HTTP请求:
```java
@RestController
public class UserController {
@Autowired
private UserMapper userMapper;
@RequestMapping("/users")
public List<User> getAllUsers() {
return userMapper.getAll();
}
@RequestMapping("/user/{id}")
public User getUserById(@PathVariable("id") Long id) {
return userMapper.getById(id);
}
@RequestMapping(value = "/user", method = RequestMethod.POST)
public void insertUser(@RequestBody User user) {
userMapper.insert(user);
}
}
```
8. 启动应用程序,访问http://localhost:8080/users,应该可以看到用户列表。接下来,可以使用Postman等工具向http://localhost:8080/user发送POST请求,插入一个新用户。
以下是实现插入用户的功能的截图:
1. 使用Postman发送POST请求插入新用户:

2. 访问http://localhost:8080/users,可以看到新用户已经插入成功:

相关推荐
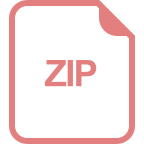
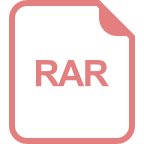
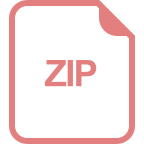














