springboot+thymeleaf+mongodb员工管理系统
时间: 2023-06-29 22:08:19 浏览: 77
好的,下面是一个简单的基于Spring Boot、Thymeleaf和MongoDB的员工管理系统的实现:
1. 首先,需要引入相关依赖。在项目的pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot web starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Spring Boot MongoDB starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
</dependencies>
```
2. 创建Employee类表示员工实体,包括姓名、年龄、职位等属性,以及相应的getter和setter方法。例如:
```java
public class Employee {
private String id;
private String name;
private int age;
private String position;
// getter and setter methods
}
```
3. 创建EmployeeRepository接口继承MongoRepository,用于操作MongoDB数据库中的Employee集合。例如:
```java
public interface EmployeeRepository extends MongoRepository<Employee, String> {
// 根据名称查询员工
List<Employee> findByName(String name);
// 根据职位查询员工
List<Employee> findByPosition(String position);
}
```
4. 创建EmployeeController类作为员工管理系统的控制器,处理员工信息的增删改查等操作。例如:
```java
@Controller
public class EmployeeController {
@Autowired
private EmployeeRepository repository;
// 查询所有员工
@GetMapping("/")
public String index(Model model) {
List<Employee> employees = repository.findAll();
model.addAttribute("employees", employees);
return "index";
}
// 新增员工
@GetMapping("/add")
public String add(Model model) {
model.addAttribute("employee", new Employee());
return "add";
}
@PostMapping("/add")
public String add(Employee employee) {
repository.save(employee);
return "redirect:/";
}
// 修改员工信息
@GetMapping("/edit/{id}")
public String edit(@PathVariable String id, Model model) {
Employee employee = repository.findById(id).orElse(null);
model.addAttribute("employee", employee);
return "edit";
}
@PostMapping("/edit")
public String edit(Employee employee) {
repository.save(employee);
return "redirect:/";
}
// 删除员工
@GetMapping("/delete/{id}")
public String delete(@PathVariable String id) {
repository.deleteById(id);
return "redirect:/";
}
// 查询员工
@PostMapping("/search")
public String search(String keyword, Model model) {
List<Employee> employees = repository.findByNameOrPosition(keyword, keyword);
model.addAttribute("employees", employees);
return "index";
}
}
```
5. 创建Thymeleaf模板,实现员工信息的展示、新增、修改和删除等操作。例如:
index.html
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Employee Management System</title>
</head>
<body>
<h1>Employee Management System</h1>
<p><a th:href="@{/add}">Add Employee</a></p>
<form th:action="@{/search}" method="post">
<input type="text" name="keyword" placeholder="Search by Name or Position"/>
<button type="submit">Search</button>
</form>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
<th>Position</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr th:each="employee : ${employees}">
<td th:text="${employee.id}"></td>
<td th:text="${employee.name}"></td>
<td th:text="${employee.age}"></td>
<td th:text="${employee.position}"></td>
<td>
<a th:href="@{/edit/{id}(id=${employee.id})}">Edit</a>
<a th:href="@{/delete/{id}(id=${employee.id})}">Delete</a>
</td>
</tr>
</tbody>
</table>
</body>
</html>
```
add.html
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Add Employee</title>
</head>
<body>
<h1>Add Employee</h1>
<form th:action="@{/add}" method="post">
<div>
<label>Name:</label>
<input type="text" name="name"/>
</div>
<div>
<label>Age:</label>
<input type="number" name="age"/>
</div>
<div>
<label>Position:</label>
<input type="text" name="position"/>
</div>
<button type="submit">Save</button>
</form>
</body>
</html>
```
edit.html
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Edit Employee</title>
</head>
<body>
<h1>Edit Employee</h1>
<form th:action="@{/edit}" method="post">
<input type="hidden" name="id" th:value="${employee.id}"/>
<div>
<label>Name:</label>
<input type="text" name="name" th:value="${employee.name}"/>
</div>
<div>
<label>Age:</label>
<input type="number" name="age" th:value="${employee.age}"/>
</div>
<div>
<label>Position:</label>
<input type="text" name="position" th:value="${employee.position}"/>
</div>
<button type="submit">Save</button>
</form>
</body>
</html>
```
6. 最后,配置MongoDB数据库连接。在application.properties文件中添加以下配置:
```properties
spring.data.mongodb.uri=mongodb://localhost:27017/employee
```
其中,employee为MongoDB数据库名称。
至此,一个基于Spring Boot、Thymeleaf和MongoDB的员工管理系统就完成了。可以使用浏览器访问http://localhost:8080/来体验该系统。
相关推荐
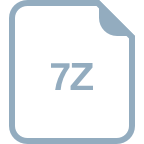
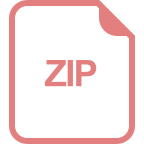
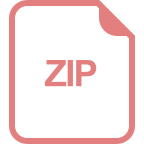









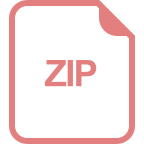
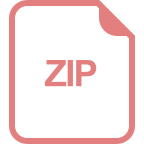
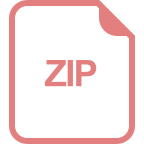
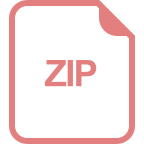
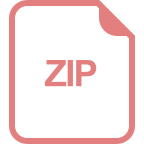
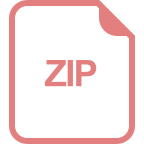