写一个王道顺序表动态数组并写上注释
时间: 2024-06-03 20:08:01 浏览: 10
// 定义一个王道顺序表动态数组类
template <typename T>
class ArrayList {
private:
T *data; // 存储数据的数组
int capacity; // 数组容量
int size; // 数组元素个数
public:
// 构造函数
ArrayList(int capacity = 10) {
this->capacity = capacity;
this->size = 0;
this->data = new T[capacity];
}
// 析构函数
~ArrayList() {
delete[] data;
}
// 获取数组元素个数
int getSize() const {
return size;
}
// 获取数组容量
int getCapacity() const {
return capacity;
}
// 判断数组是否为空
bool isEmpty() const {
return size == 0;
}
// 在数组末尾添加元素
void addLast(T e) {
add(size, e);
}
// 在数组开头添加元素
void addFirst(T e) {
add(0, e);
}
// 在指定位置添加元素
void add(int index, T e) {
if (index < 0 || index > size) {
throw std::out_of_range("Index out of range");
}
if (size == capacity) {
resize(capacity * 2);
}
// 将 index 及其后面的元素后移一位
for (int i = size - 1; i >= index; i--) {
data[i + 1] = data[i];
}
data[index] = e;
size++;
}
// 获取指定位置的元素
T get(int index) const {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
// 设置指定位置的元素
void set(int index, T e) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
data[index] = e;
}
// 判断数组是否包含指定元素
bool contains(T e) const {
for (int i = 0; i < size; i++) {
if (data[i] == e) {
return true;
}
}
return false;
}
// 查找指定元素的索引,若不存在返回 -1
int find(T e) const {
for (int i = 0; i < size; i++) {
if (data[i] == e) {
return i;
}
}
return -1;
}
// 删除指定位置的元素
T remove(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
T ret = data[index];
// 将 index 及其后面的元素前移一位
for (int i = index + 1; i < size; i++) {
data[i - 1] = data[i];
}
size--;
// 缩小数组容量
if (size == capacity / 4 && capacity / 2 != 0) {
resize(capacity / 2);
}
return ret;
}
// 删除第一个元素
T removeFirst() {
return remove(0);
}
// 删除最后一个元素
T removeLast() {
return remove(size - 1);
}
// 删除指定元素,若不存在则不做处理
void removeElement(T e) {
int index = find(e);
if (index != -1) {
remove(index);
}
}
// 将数组容量改变为 newCapacity
void resize(int newCapacity) {
T *newData = new T[newCapacity];
for (int i = 0; i < size; i++) {
newData[i] = data[i];
}
data = newData;
capacity = newCapacity;
}
// 重载输出运算符
friend std::ostream& operator<<(std::ostream& os, const ArrayList<T>& arr) {
os << "ArrayList: size = " << arr.size << ", capacity = " << arr.capacity << std::endl;
os << "[";
for (int i = 0; i < arr.size; i++) {
os << arr.data[i];
if (i != arr.size - 1) {
os << ", ";
}
}
os << "]";
return os;
}
};
相关推荐
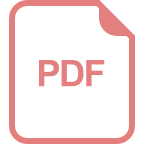
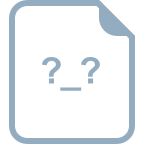













