import re str1 = '123hello python!123' str2 = re.findall('123(.*)123', str1)[0] print(str2)
时间: 2024-01-24 20:01:36 浏览: 25
这段代码的作用是从字符串 `str1` 中提取出两个 "123" 之间的内容,即 "hello python!",然后将其打印出来。
具体来说,`re.findall()` 函数是 Python 中的正则表达式模块 `re` 中的一个函数,它可以匹配字符串中所有符合指定模式的子串,并返回一个列表。在这里,模式为 `'123(.*)123'`,其中 `.*` 表示匹配任意字符(除了换行符)0 次或多次,括号中的 `.*` 表示将匹配到的内容作为一个组来保存。
因此,`re.findall('123(.*)123', str1)` 的返回值为一个列表,其中只有一个元素,即 `['hello python!']`。由于我们只需要提取这个元素,所以加上 `[0]` 将其提取出来,并将其赋值给变量 `str2`。最后,将 `str2` 打印出来即可。
相关问题
如何将这段代码import re print("开始提取字符串") run = ".*" + start + "(.*)" + end + ".*" result = re.findall(run, str) for str_extraction in result: print(str_extraction)的str_extraction值得返回出来
你可以将`str_extraction`添加到一个列表中,并在函数末尾使用`return`语句将该列表返回。例如:
```python
import re
def extract_string(str, start, end):
print("开始提取字符串")
run = ".*" + start + "(.*)" + end + ".*"
result = re.findall(run, str)
extractions = []
for str_extraction in result:
extractions.append(str_extraction)
print(str_extraction)
return extractions
```
这样,当你调用`extract_string`函数时,它将返回一个包含所有提取的字符串的列表。你可以将该列表赋值给一个变量,然后在需要时使用。例如:
```python
my_str = "Hello World! This is a test string. Let's extract some text."
start = "This is "
end = " string."
extractions = extract_string(my_str, start, end)
print(extractions)
```
这将输出:
```
开始提取字符串
['a test']
```
python str find 从后往前找
Python中的字符串方法`find()`可以从前往后查找子字符串,如果要从后往前找,可以使用`rfind()`方法。`rfind()`的用法与`find()`相同,只不过是从字符串的末尾开始查找。例如:
```
s = "hello world"
print(s.rfind("l")) # 输出9,即最后一个字符"l"的索引
```
如果要查找所有匹配的子字符串,可以使用正则表达式或者`re`模块的`findall()`方法。例如:
```python
import re
s = "hello world"
matches = re.findall("l", s)
print(matches) # 输出['l', 'l']
```
这样可以找到所有的字符"l",而不仅仅是最后一个。
相关推荐
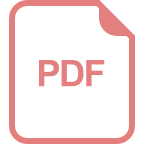
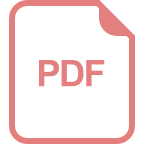
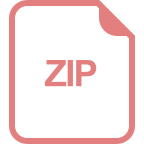












