JAVA word转pdf代码
时间: 2023-07-27 18:06:05 浏览: 47
以下是使用 Apache POI 和 iText 库将 Word 转为 PDF 的 Java 代码:
```java
import java.io.*;
import org.apache.poi.xwpf.usermodel.*;
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.*;
public class WordToPdfConverter {
public static void main(String[] args) throws Exception {
// 读取 Word 文档
FileInputStream fis = new FileInputStream(new File("input.docx"));
XWPFDocument document = new XWPFDocument(fis);
// 创建 PDF 文档
Document pdfDocument = new Document();
FileOutputStream fos = new FileOutputStream(new File("output.pdf"));
PdfWriter.getInstance(pdfDocument, fos);
pdfDocument.open();
// 遍历 Word 文档中的段落和表格
for (IBodyElement element : document.getBodyElements()) {
if (element instanceof XWPFParagraph) {
// 处理段落
XWPFParagraph paragraph = (XWPFParagraph) element;
Paragraph pdfParagraph = new Paragraph(paragraph.getText());
pdfDocument.add(pdfParagraph);
} else if (element instanceof XWPFTable) {
// 处理表格
XWPFTable table = (XWPFTable) element;
PdfPTable pdfTable = new PdfPTable(table.getNumberOfColumns());
for (int i = 0; i < table.getNumberOfRows(); i++) {
XWPFTableRow row = table.getRow(i);
for (int j = 0; j < row.getTableCells().size(); j++) {
XWPFTableCell cell = row.getCell(j);
PdfPCell pdfCell = new PdfPCell(new Phrase(cell.getText()));
pdfTable.addCell(pdfCell);
}
}
pdfDocument.add(pdfTable);
}
}
// 关闭文档
pdfDocument.close();
fis.close();
fos.close();
}
}
```
请将 `input.docx` 替换为你的 Word 文档文件名,将 `output.pdf` 替换为你想要生成的 PDF 文件名。此外,你需要将 Apache POI 和 iText 库添加到你的项目中以使代码编译通过。
相关推荐
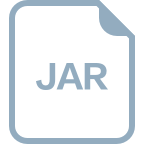







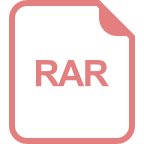
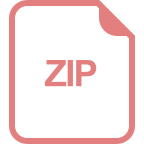
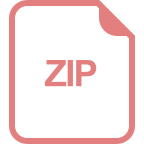
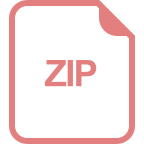