实现“焦点轮播图”效果,共有01.jpg,02.jpg,03.jpg三张图片,要求如下:(1)当页面加载完成后,每2秒自动切换一张图片(2)切换图片时,其对应按钮的样式同步变换——背景颜色变成红色(3)当鼠标悬停在某个按钮上时,显示该按钮对应的图片且轮播停止
时间: 2023-12-04 14:06:03 浏览: 73
好的,这是一个比较常见的前端开发问题,你可以按照以下步骤实现:
1. HTML结构
首先,你需要在HTML中添加一个父元素用于容纳轮播图和对应的按钮,同时需要添加相应的图片和按钮元素,具体代码如下:
```html
<div class="carousel">
<img src="01.jpg" alt="image1">
<img src="02.jpg" alt="image2">
<img src="03.jpg" alt="image3">
<div class="btn-group">
<button class="btn active"></button>
<button class="btn"></button>
<button class="btn"></button>
</div>
</div>
```
2. CSS样式
接下来,你需要为轮播图和按钮添加相应的CSS样式,具体代码如下:
```css
.carousel {
position: relative;
width: 600px;
height: 400px;
overflow: hidden;
}
.carousel img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 1s ease-in-out;
}
.carousel img.active {
opacity: 1;
}
.btn-group {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
.btn {
display: inline-block;
width: 10px;
height: 10px;
margin: 0 10px;
background-color: #ccc;
border-radius: 50%;
cursor: pointer;
}
.btn.active {
background-color: red;
}
```
3. JavaScript代码
最后,你需要编写JavaScript代码来实现轮播图的自动播放和按钮的切换,具体代码如下:
```javascript
var carousel = document.querySelector('.carousel');
var images = carousel.querySelectorAll('img');
var buttons = carousel.querySelectorAll('.btn');
var currentIndex = 0;
var timer;
// 自动播放
function play() {
timer = setInterval(function() {
var lastIndex = currentIndex;
currentIndex = (currentIndex + 1) % images.length;
images[lastIndex].classList.remove('active');
images[currentIndex].classList.add('active');
buttons[lastIndex].classList.remove('active');
buttons[currentIndex].classList.add('active');
}, 2000);
}
// 停止播放
function stop() {
clearInterval(timer);
}
// 切换图片
function moveTo(index) {
var lastIndex = currentIndex;
currentIndex = index;
images[lastIndex].classList.remove('active');
images[currentIndex].classList.add('active');
buttons[lastIndex].classList.remove('active');
buttons[currentIndex].classList.add('active');
}
// 绑定事件
carousel.addEventListener('mouseover', function() {
stop();
});
carousel.addEventListener('mouseout', function() {
play();
});
buttons.forEach(function(button, index) {
button.addEventListener('click', function() {
moveTo(index);
});
});
// 启动轮播
play();
```
这样,你就可以实现一个简单的焦点轮播图效果了。
阅读全文
相关推荐
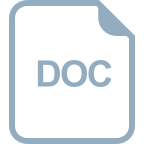
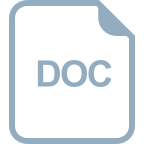
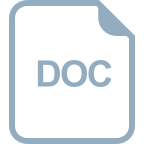


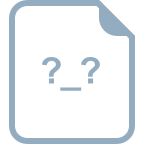
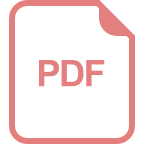
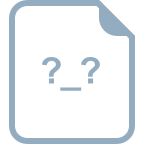
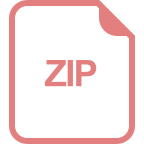
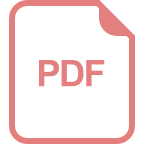
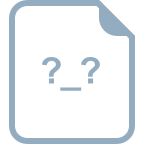
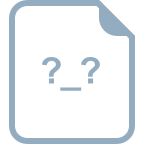
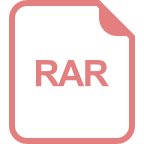
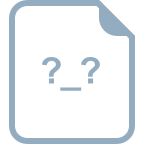
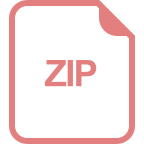
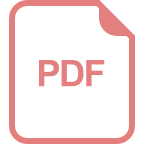


