log_attention_weights = False
时间: 2023-12-20 13:06:23 浏览: 70
这段代码是给变量log_attention_weights赋值为False。具体来说,这个变量可能是用来控制是否将注意力权重的对数输出到日志中。在深度学习中,注意力机制被广泛应用于各种任务,例如机器翻译、语音识别、自然语言处理等。注意力权重表示了模型对输入数据中不同部分的关注程度,因此可以用来解释模型的预测结果和行为。将注意力权重的对数输出到日志中可以帮助我们更好地理解模型的内部机制,但同时也会增加计算和存储的开销。
相关问题
写出下面完整的程序:pytorch实现时序预测,用lstm、attention、encoder-decoder和Knowledge Distillation四种技术。注意是完整的代码
import torch
from torch import nn
from torch.nn import functional as F# 定义encoder
class Encoder(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, dropout=0, bidirectional=False):
super(Encoder, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.bidirectional = bidirectional
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, dropout=dropout, bidirectional=bidirectional)
def forward(self, x, hidden):
output, hidden = self.lstm(x, hidden)
return output, hidden
def init_hidden(self, batch_size):
num_directions = 2 if self.bidirectional else 1
h0 = torch.zeros(self.num_layers * num_directions, batch_size, self.hidden_size)
c0 = torch.zeros(self.num_layers * num_directions, batch_size, self.hidden_size)
return (h0, c0)# 定义Attention
class Attention(nn.Module):
def __init__(self, hidden_size):
super(Attention, self).__init__()
self.hidden_size = hidden_size
self.attn = nn.Linear(self.hidden_size * 2, hidden_size)
self.v = nn.Parameter(torch.rand(hidden_size))
stdv = 1. / math.sqrt(self.v.size(0))
self.v.data.normal_(mean=0, std=stdv)
def forward(self, hidden, encoder_outputs):
# hidden: [batch_size, hidden_size]
# encoder_outputs: [seq_len, batch_size, hidden_size * 2]
seq_len = encoder_outputs.size(0)
# repeat hidden
hidden = hidden.unsqueeze(1).repeat(1, seq_len, 1)
encoder_outputs = encoder_outputs.permute(1, 0, 2)
# hidden: [batch_size, seq_len, hidden_size]
# encoder_outputs: [batch_size, seq_len, hidden_size * 2]
energy = torch.tanh(self.attn(torch.cat([hidden, encoder_outputs], 2)))
# energy: [batch_size, seq_len, hidden_size]
energy = energy.permute(0, 2, 1)
# v: [hidden_size]
v = self.v.repeat(encoder_outputs.size(0), 1).unsqueeze(1)
# v: [batch_size, 1, hidden_size]
# attn_weights: [batch_size, seq_len]
attn_weights = torch.bmm(v, energy).squeeze(1)
return F.softmax(attn_weights, dim=1).unsqueeze(1)# 定义Decoder
class Decoder(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, dropout=0):
super(Decoder, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.attention = Attention(hidden_size)
self.lstm = nn.LSTM(input_size + hidden_size, hidden_size, num_layers, dropout=dropout)
self.out = nn.Linear(hidden_size, input_size)
def forward(self, x, hidden, encoder_outputs):
# x: [batch_size]
# hidden: [num_layers, batch_size, hidden_size]
# encoder_outputs: [seq_len, batch_size, hidden_size * 2]
x = x.unsqueeze(1)
# x: [batch_size, 1]
x = F.relu(self.out(x))
# x: [batch_size, 1, input_size]
seq_len = encoder_outputs.size(0)
context = self.attention(hidden[-1], encoder_outputs)
# context: [batch_size, 1, seq_len]
context = context.repeat(1, x.size(1), 1)
# context: [batch_size, 1, seq_len]
x = torch.cat([x, context], 2)
# x: [batch_size, 1, input_size + seq_len]
output, hidden = self.lstm(x, hidden)
output = output.squeeze(1)
output = F.log_softmax(self.out(output), dim=1)
return output, hidden, context# 定义Knowledge Distillation
class KnowledgeDistillation(nn.Module):
def __init__(self, teacher_model, student_model):
super(KnowledgeDistillation, self).__init__()
self.teacher_model = teacher_model
self.student_model = student_model
def forward(self, x, targets):
teacher_logits, student_logits = self.teacher_model(x), self.student_model(x)
loss = F.kl_div(F.log_softmax(student_logits, dim=1), F.softmax(teacher_logits / 5, dim=1))
distillation_loss = F.cross_entropy(student_logits, targets) + loss
return distillation_loss
阅读全文
相关推荐
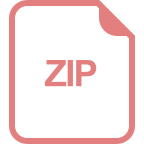
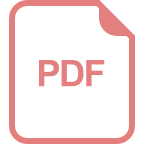
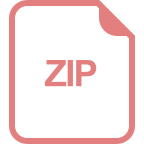
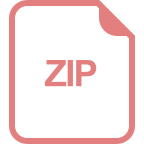
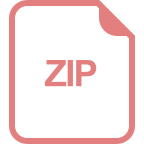
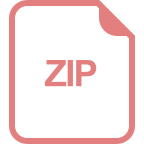
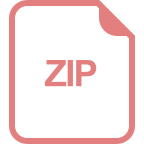
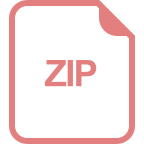
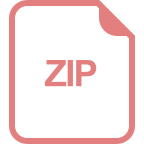
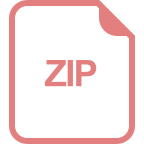
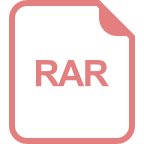
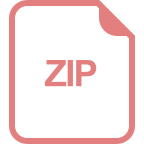