web服务器c语言加载图片
时间: 2023-08-24 07:12:57 浏览: 53
要在web服务器中使用C语言加载图像,你需要使用一个库来处理图像,例如OpenCV或ImageMagick。这些库可以帮助你读取和解码各种图像格式,并将它们转换为可以在web页面上显示的格式,例如JPEG或PNG。
下面是一个使用OpenCV库加载JPEG图像并将其作为响应发送给客户端的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <opencv2/opencv.hpp>
int main(int argc, char **argv) {
// Read image file
cv::Mat img = cv::imread("image.jpg", cv::IMREAD_COLOR);
// Check if image was loaded successfully
if (img.empty()) {
printf("Failed to load image file\n");
return 1;
}
// Encode image as JPEG
std::vector<uchar> buf;
std::vector<int> param = {cv::IMWRITE_JPEG_QUALITY, 90};
cv::imencode(".jpg", img, buf, param);
// Prepare response headers
const char *response_headers =
"HTTP/1.1 200 OK\r\n"
"Content-Type: image/jpeg\r\n"
"Content-Length: %lu\r\n"
"\r\n";
// Calculate content length
size_t content_length = buf.size();
// Allocate response buffer
char *response_buf = (char *) malloc(strlen(response_headers) + 64 + content_length);
// Write response headers
sprintf(response_buf, response_headers, content_length);
// Write image data
memcpy(response_buf + strlen(response_headers), buf.data(), content_length);
// Send response
fwrite(response_buf, strlen(response_headers) + content_length, 1, stdout);
// Cleanup
free(response_buf);
return 0;
}
```
这个示例读取名为“image.jpg”的JPEG图像文件,将其编码为JPEG格式,并将其作为响应发送给客户端。请注意,这只是一个简单的示例代码,你需要根据你的具体需求进行修改。
相关推荐
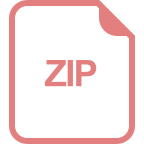
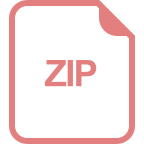
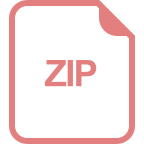














