ios php 推送消息,用PHP实现IOS推送
时间: 2024-03-26 22:34:48 浏览: 106
要实现iOS推送,需要遵循以下步骤:
1. 创建APNS证书
首先,你需要在苹果开发者中心创建一个APNS证书。这个证书将用于安全地将消息发送到iOS设备。
2. 获取设备Token
每个iOS设备都有一个唯一的设备Token,用于标识该设备。你需要在你的应用中获取该设备Token,并将其发送到你的服务器。
3. 编写PHP代码
使用PHP编写代码,以便将消息发送到APNS服务器。你需要使用APNS证书和设备Token来建立连接,并将消息发送到APNS服务器。
以下是一个简单的PHP代码示例:
```
<?php
// Put your device token here (without spaces):
$deviceToken = 'YOUR_DEVICE_TOKEN_HERE';
// Put your private key's passphrase here:
$passphrase = 'YOUR_PASSPHRASE_HERE';
// Put your alert message here:
$message = 'Hello, world!';
////////////////////////////////////////////////////////////////////////////////
$ctx = stream_context_create();
stream_context_set_option($ctx, 'ssl', 'local_cert', 'YOUR_APNS_CERTIFICATE.pem');
stream_context_set_option($ctx, 'ssl', 'passphrase', $passphrase);
// Open a connection to the APNS server
$fp = stream_socket_client(
'ssl://gateway.sandbox.push.apple.com:2195', $err,
$errstr, 60, STREAM_CLIENT_CONNECT|STREAM_CLIENT_PERSISTENT, $ctx);
if (!$fp)
exit("Failed to connect: $err $errstr" . PHP_EOL);
echo 'Connected to APNS' . PHP_EOL;
// Create the payload body
$body['aps'] = array(
'alert' => $message,
'sound' => 'default'
);
// Encode the payload as JSON
$payload = json_encode($body);
// Build the binary notification
$msg = chr(0) . pack('n', 32) . pack('H*', $deviceToken) . pack('n', strlen($payload)) . $payload;
// Send the notification to the server
$result = fwrite($fp, $msg, strlen($msg));
if (!$result)
echo 'Message not delivered' . PHP_EOL;
else
echo 'Message successfully delivered' . PHP_EOL;
// Close the connection to the server
fclose($fp);
?>
```
在上面的代码中,你需要将以下变量替换为你自己的值:
- YOUR_DEVICE_TOKEN_HERE:你的设备Token。
- YOUR_PASSPHRASE_HERE:你的APNS证书密码。
- YOUR_APNS_CERTIFICATE.pem:你的APNS证书文件名和路径。
- $message:你要发送的消息。
4. 测试推送
运行PHP代码并测试推送。如果一切正常,你应该会收到一个推送通知。
注意:在生产环境中,你需要将APNS服务器地址更改为“gateway.push.apple.com”。在示例代码中,我们使用的是开发环境的APNS服务器地址“gateway.sandbox.push.apple.com”。
阅读全文
相关推荐
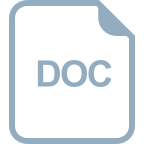
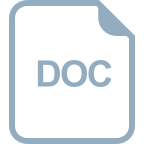
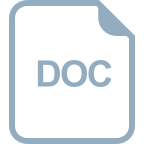
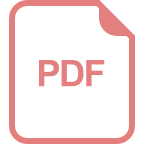
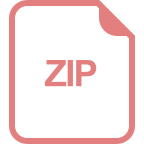
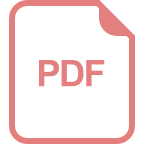
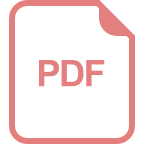
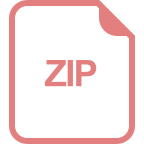
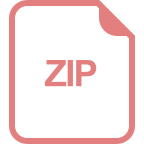
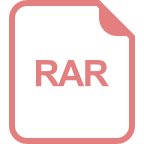
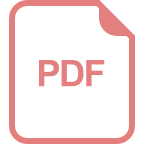
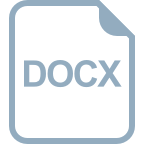
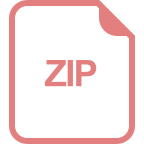
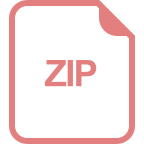
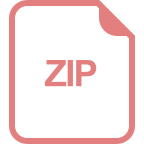
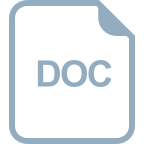
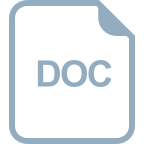