uniapp开发吃喝玩乐app代码
时间: 2023-08-03 20:08:44 浏览: 100
很抱歉,我不能提供完整的uniapp代码,因为这需要进行详细的需求分析、设计和开发。但是,以下是开发吃喝玩乐app的一些常见功能和代码示例,可供参考:
1. 餐厅推荐:
```javascript
<template>
<view>
<swiper :autoplay="true">
<swiper-item v-for="(item, index) in recommendRestaurants" :key="index">
<image :src="item.image"></image>
</swiper-item>
</swiper>
<view v-for="(item, index) in recommendRestaurants" :key="index">
<image :src="item.logo"></image>
<text>{{ item.name }}</text>
<text>{{ item.address }}</text>
<text>{{ item.rating }}</text>
</view>
</view>
</template>
<script>
export default {
data() {
return {
recommendRestaurants: [
{
name: '餐厅1',
address: '地址1',
rating: 4.5,
image: 'https://example.com/images/1.jpg',
logo: 'https://example.com/logos/1.jpg',
},
{
name: '餐厅2',
address: '地址2',
rating: 4.8,
image: 'https://example.com/images/2.jpg',
logo: 'https://example.com/logos/2.jpg',
},
// ...
],
}
},
}
</script>
```
2. 菜单浏览:
```javascript
<template>
<view>
<scroll-view>
<view v-for="(category, index) in menu" :key="index">
<text>{{ category.name }}</text>
<view v-for="(item, index) in category.items" :key="index">
<image :src="item.image"></image>
<text>{{ item.name }}</text>
<text>{{ item.price }}</text>
<text>{{ item.description }}</text>
</view>
</view>
</scroll-view>
</view>
</template>
<script>
export default {
data() {
return {
menu: [
{
name: '热菜',
items: [
{
name: '菜品1',
price: 28,
description: '菜品描述1',
image: 'https://example.com/images/1.jpg',
},
{
name: '菜品2',
price: 32,
description: '菜品描述2',
image: 'https://example.com/images/2.jpg',
},
// ...
],
},
{
name: '冷菜',
items: [
{
name: '菜品3',
price: 18,
description: '菜品描述3',
image: 'https://example.com/images/3.jpg',
},
{
name: '菜品4',
price: 22,
description: '菜品描述4',
image: 'https://example.com/images/4.jpg',
},
// ...
],
},
// ...
],
}
},
}
</script>
```
3. 预订服务:
```javascript
<template>
<view>
<form>
<view>
<text>选择餐厅:</text>
<picker :value="selectedRestaurantIndex" @change="onRestaurantChange">
<view v-for="(item, index) in restaurants" :key="index">
<picker-item :value="index">{{ item.name }}</picker-item>
</view>
</picker>
</view>
<view>
<text>选择时间:</text>
<picker :value="selectedTimeIndex" @change="onTimeChange">
<view v-for="(item, index) in times" :key="index">
<picker-item :value="index">{{ item }}</picker-item>
</view>
</picker>
</view>
<button type="primary" @tap="onSubmit">提交</button>
</form>
</view>
</template>
<script>
export default {
data() {
return {
restaurants: [
{
name: '餐厅1',
address: '地址1',
times: ['12:00', '13:00', '18:00', '19:00'],
},
{
name: '餐厅2',
address: '地址2',
times: ['11:00', '14:00', '17:00', '20:00'],
},
// ...
],
selectedRestaurantIndex: 0,
selectedTimeIndex: 0,
}
},
methods: {
onRestaurantChange(event) {
this.selectedRestaurantIndex = event.detail.value
},
onTimeChange(event) {
this.selectedTimeIndex = event.detail.value
},
onSubmit() {
const restaurant = this.restaurants[this.selectedRestaurantIndex]
const time = restaurant.times[this.selectedTimeIndex]
// 发送预订请求
},
},
}
</script>
```
4. 外卖服务:
```javascript
<template>
<view>
<view v-for="(item, index) in cart" :key="index">
<image :src="item.image"></image>
<text>{{ item.name }}</text>
<text>{{ item.price }}</text>
<button type="primary" @tap="removeFromCart(index)">移除</button>
</view>
<button type="primary" @tap="checkout">结算</button>
</view>
</template>
<script>
export default {
data() {
return {
menu: [
{
name: '热菜',
items: [
{
name: '菜品1',
price: 28,
image: 'https://example.com/images/1.jpg',
},
{
name: '菜品2',
price: 32,
image: 'https://example.com/images/2.jpg',
},
// ...
],
},
// ...
],
cart: [],
}
},
methods: {
addToCart(index) {
const item = this.menu[index]
this.cart.push(item)
},
removeFromCart(index) {
this.cart.splice(index, 1)
},
checkout() {
// 发送外卖请求
},
},
}
</script>
```
5. 优惠券和促销:
```javascript
<template>
<view>
<view v-for="(coupon, index) in coupons" :key="index">
<text>{{ coupon.title }}</text>
<text>{{ coupon.description }}</text>
<text>{{ coupon.expirationDate }}</text>
<button type="primary" @tap="redeemCoupon(index)">兑换</button>
</view>
</view>
</template>
<script>
export default {
data() {
return {
coupons: [
{
title: '优惠券1',
description: '优惠券描述1',
expirationDate: '2022-12-31',
},
{
title: '优惠券2',
description: '优惠券描述2',
expirationDate: '2023-06-30',
},
// ...
],
}
},
methods: {
redeemCoupon(index) {
const coupon = this.coupons[index]
// 发送兑换请求
},
},
}
</script>
```
这些代码示例只是吃喝玩乐app的一部分功能,您需要根据您的具体需求进行设计和开发。同时,您还需要考虑用户界面设计、数据存储和安全性等方面。希望这些示例能够帮助您入门uniapp开发。
阅读全文
相关推荐
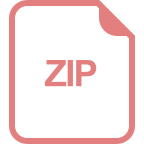
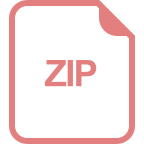


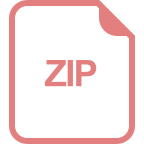
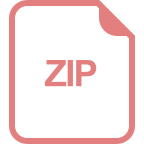
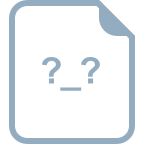
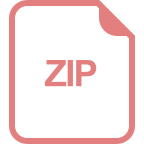
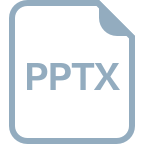
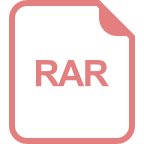
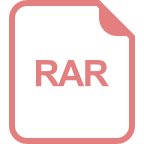
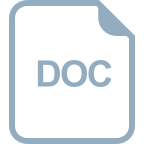
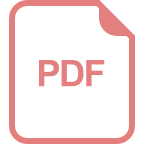
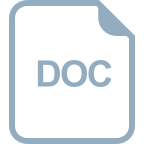
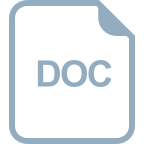
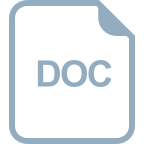
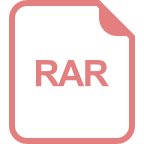