用户生日存放在wstring中,读取系统时间,判断今天是否是用户的生日 C++ 不使用localtime()
时间: 2024-02-23 10:01:38 浏览: 60
如果不使用 `localtime()` 函数,可以使用 C++11 中的 `<chrono>` 库来获取当前系统时间,并使用 `<regex>` 库来从 `wstring` 中提取出生日的月份和日期。以下是一个实现示例:
```c++
#include <chrono>
#include <iostream>
#include <regex>
#include <string>
int main() {
// 获取当前时间
auto now = std::chrono::system_clock::now();
auto now_time = std::chrono::system_clock::to_time_t(now);
std::tm* time = std::localtime(&now_time);
int currentMonth = time->tm_mon + 1;
int currentDay = time->tm_mday;
// 从 wstring 中读取用户生日
std::wstring userBirth = L"1990年12月25日"; // 这里假设用户生日是 1990 年 12 月 25 日
std::wregex pattern(L"([0-9]{2})月([0-9]{2})日");
std::wsmatch match;
if (std::regex_search(userBirth, match, pattern)) {
int birthMonth = std::stoi(match[1]);
int birthDay = std::stoi(match[2]);
// 判断今天是否是用户的生日
if (currentMonth == birthMonth && currentDay == birthDay) {
std::wcout << L"今天是你的生日,祝你生日快乐!" << std::endl;
} else {
std::wcout << L"今天不是你的生日。" << std::endl;
}
}
return 0;
}
```
在这个代码中,我们使用 `std::chrono::system_clock::now()` 函数获取当前系统时间,并使用 `std::chrono::system_clock::to_time_t()` 函数将时间转换为 `time_t` 类型。然后,我们使用 `std::localtime()` 函数将时间转换为 `std::tm` 结构体。接下来,我们使用正则表达式从 `wstring` 中提取出生日的月份和日期。最后,我们将当前的月份和日期与用户的生日进行比较,如果相同,则输出生日快乐的祝福,否则输出其它内容。需要注意的是,我们需要在输出中使用 `std::wcout` 来输出 Unicode 字符串。
阅读全文
相关推荐
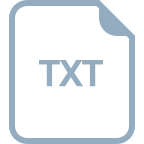















