c语言代码实现位图直方图均衡,编写一个程序,可以在命令行输入参数,完成指定文件的直方图均衡,并存储到新文件,命令行参数如下 hist file1.bmp file2.bmp
时间: 2024-03-08 18:47:42 浏览: 86
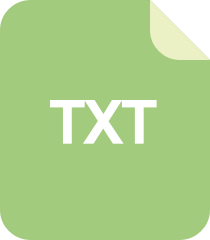
直方图均衡C语言程序
好的,以下是 C 语言代码实现直方图均衡的示例,您可以在命令行中输入 "hist file1.bmp file2.bmp" 来进行图像处理。其中 file1.bmp 是您要进行直方图均衡的源文件名,file2.bmp 是您要保存结果的目标文件名。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#pragma pack(2)
typedef struct {
uint16_t type;
uint32_t size;
uint16_t reserved1;
uint16_t reserved2;
uint32_t offset;
} BMPHeader;
#pragma pack(2)
typedef struct {
uint32_t size;
int32_t width;
int32_t height;
uint16_t planes;
uint16_t bits_per_pixel;
uint32_t compression;
uint32_t image_size;
int32_t x_pixels_per_meter;
int32_t y_pixels_per_meter;
uint32_t colors_used;
uint32_t colors_important;
} BMPInfo;
void histogram_equalization(uint8_t* image, int width, int height) {
int histogram[256] = { 0 };
int cdf[256] = { 0 };
int cumulative = 0;
// 统计每个像素值的出现次数
for (int i = 0; i < width * height; i++) {
histogram[image[i]]++;
}
// 计算累计分布函数
for (int i = 0; i < 256; i++) {
cumulative += histogram[i];
cdf[i] = cumulative;
}
// 直方图均衡化
for (int i = 0; i < width * height; i++) {
int pixel = image[i];
int new_pixel = 255 * cdf[pixel] / (width * height);
image[i] = new_pixel;
}
}
int main(int argc, char** argv) {
if (argc != 3) {
printf("Usage: hist <source_file> <target_file>\n");
return 0;
}
char* source_file = argv[1];
char* target_file = argv[2];
FILE* fp = fopen(source_file, "rb");
if (!fp) {
printf("Failed to open source file\n");
return 0;
}
BMPHeader header;
BMPInfo info;
fread(&header, sizeof(header), 1, fp);
fread(&info, sizeof(info), 1, fp);
if (header.type != 0x4d42 || info.bits_per_pixel != 8) {
printf("Unsupported image format\n");
fclose(fp);
return 0;
}
int width = abs(info.width);
int height = abs(info.height);
int padding = (4 - ((width * 8) % 32) / 8) % 4;
uint8_t* image = (uint8_t*)malloc(width * height);
fread(image, 1, width * height, fp);
fclose(fp);
histogram_equalization(image, width, height);
fp = fopen(target_file, "wb");
if (!fp) {
printf("Failed to save target file\n");
free(image);
return 0;
}
fwrite(&header, sizeof(header), 1, fp);
fwrite(&info, sizeof(info), 1, fp);
fwrite(image, 1, width * height, fp);
uint8_t pad[4] = { 0 };
for (int i = 0; i < padding; i++) {
fwrite(pad, 1, 4, fp);
}
fclose(fp);
free(image);
printf("Histogram equalization completed\n");
return 0;
}
```
该程序使用了标准 C 库函数和文件操作函数,读取和保存图像文件,以及进行直方图均衡化。在函数 `histogram_equalization` 中,使用了与 Python 版本相同的直方图统计、累计分布函数计算和直方图均衡化算法。程序中还包括了 BMP 文件头和信息头的结构体定义和读写代码,以及对图像格式的判断和错误处理。
阅读全文
相关推荐



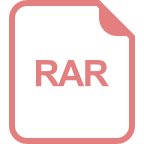
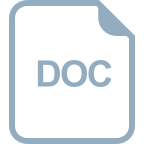
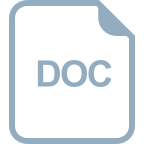
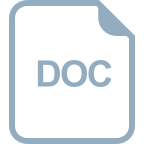

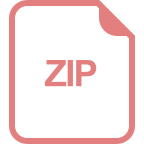
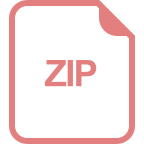
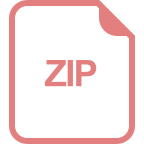
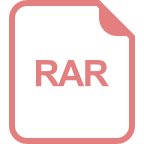
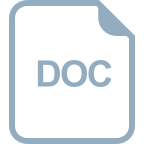
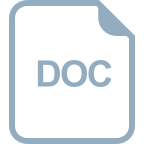
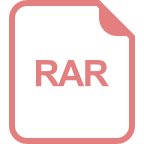