KS_means PYTHON代码 以及数据样例,聚类图
时间: 2024-06-09 10:11:52 浏览: 121
以下是使用Python实现K-means聚类算法的示例代码,以及一个数据样例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义K-means函数
def kmeans(X, k, max_iters=50):
centroids = X[np.random.choice(range(len(X)), k), :]
for i in range(max_iters):
# 计算每个样本点与聚类中心的距离
distances = np.sqrt(((X - centroids[:, np.newaxis])**2).sum(axis=2))
# 确定每个样本点所属的聚类中心
labels = np.argmin(distances, axis=0)
# 更新聚类中心
for j in range(k):
centroids[j] = X[labels==j].mean(axis=0)
return centroids, labels
# 生成数据样例
np.random.seed(42)
X = np.vstack((np.random.randn(100, 2) + np.array([5, 5]),
np.random.randn(100, 2) + np.array([-5, -5]),
np.random.randn(100, 2) + np.array([-5, 5])))
# 调用K-means函数进行聚类
centroids, labels = kmeans(X, k=3)
# 绘制聚类图
plt.figure(figsize=(8, 6))
plt.scatter(X[:, 0], X[:, 1], c=labels, s=50)
plt.scatter(centroids[:, 0], centroids[:, 1], c='red', s=200, marker='*')
plt.title('K-means Clustering')
plt.xlabel('X1')
plt.ylabel('X2')
plt.show()
```
数据样例包含300个二维数据点,其中有三个簇,每个簇的中心分别为(5,5), (-5,-5)和(-5,5)。
聚类图如下所示:

阅读全文
相关推荐






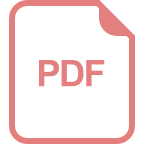
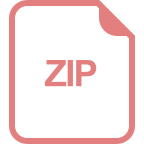
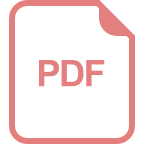
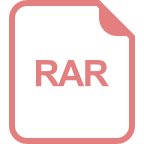
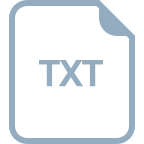
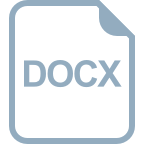