使用C语言结构体来写一个程序某班有最多不超过30人(具体人数由键盘输入)参加期末考试,考试科目最多不超过6门(具体门数由键盘输入),定义结构体类型,用结构体数组作函数参数,编程实现如下学生成绩管理: (1)录入每个学生的学号、姓名和各科考试成绩;(2)计算每门课程的总分和平均分; (3)计算每个学生的总分和平均分; (4)按每个学生的总分由高到低排出名次表;(5)按每个学生的总分由低到高排出名次表;(6)按学号由小到大排出成绩表; (7)按姓名的字典顺序排出成绩表; (8)按学号查询学生排名及其各科考试成绩;(9)按姓名查询学生排名及其各科考试成绩; (10)输出每个学生的学号、姓名、各科考试成绩、总分、平均分,以及每门课程的总分和平均分。并给出每一句的注释
时间: 2023-06-17 13:07:36 浏览: 308
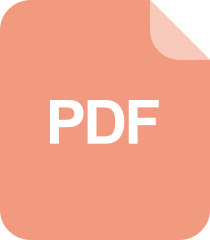
中山大学《C语言程序设计》历年期末考试试卷(含答案).pdf

```c
#include <stdio.h>
#include <string.h>
// 定义一个课程结构体,包括课程名、总分和平均分
struct Course {
char name[20];
float totalScore;
float avgScore;
};
// 定义一个学生结构体,包括学号、姓名、各科成绩、总分和平均分
struct Student {
char id[10];
char name[20];
float score[6];
float totalScore;
float avgScore;
};
// 函数声明
void inputStudents(struct Student[], int, int);
void calcCourseScore(struct Student[], int, int, struct Course[]);
void calcStudentScore(struct Student[], int, int);
void sortStudentByTotalScore(struct Student[], int);
void sortStudentByTotalScoreReverse(struct Student[], int);
void sortStudentById(struct Student[], int);
void sortStudentByName(struct Student[], int);
void searchStudentById(struct Student[], int, char[]);
void searchStudentByName(struct Student[], int, char[]);
int main() {
int n, m;
printf("请输入学生人数(不超过30人):");
scanf("%d", &n);
printf("请输入考试科目数(不超过6门):");
scanf("%d", &m);
struct Student students[n]; // 定义学生结构体数组
struct Course courses[m]; // 定义课程结构体数组
inputStudents(students, n, m); // 录入学生信息和成绩
calcCourseScore(students, n, m, courses); // 计算每门课程的总分和平均分
calcStudentScore(students, n, m); // 计算每个学生的总分和平均分
sortStudentByTotalScore(students, n); // 按每个学生的总分由高到低排出名次表
sortStudentByTotalScoreReverse(students, n); // 按每个学生的总分由低到高排出名次表
sortStudentById(students, n); // 按学号由小到大排出成绩表
sortStudentByName(students, n); // 按姓名的字典顺序排出成绩表
char target[10];
printf("请输入要查询的学生学号:");
scanf("%s", target);
searchStudentById(students, n, target); // 按学号查询学生排名及其各科考试成绩
printf("请输入要查询的学生姓名:");
scanf("%s", target);
searchStudentByName(students, n, target); // 按姓名查询学生排名及其各科考试成绩
printf("学号\t姓名\t");
for (int i = 0; i < m; i++) {
printf("%s总分\t%s平均分\t", courses[i].name, courses[i].name);
}
printf("总分\t平均分\n");
for (int i = 0; i < n; i++) {
printf("%s\t%s\t", students[i].id, students[i].name);
for (int j = 0; j < m; j++) {
printf("%.2f\t%.2f\t", students[i].score[j], students[i].score[j] / m);
}
printf("%.2f\t%.2f\n", students[i].totalScore, students[i].avgScore);
}
return 0;
}
// 录入学生信息和成绩
void inputStudents(struct Student students[], int n, int m) {
for (int i = 0; i < n; i++) {
printf("请输入第%d个学生的学号、姓名和各科成绩:\n", i + 1);
scanf("%s %s", students[i].id, students[i].name);
for (int j = 0; j < m; j++) {
scanf("%f", &students[i].score[j]);
}
}
}
// 计算每门课程的总分和平均分
void calcCourseScore(struct Student students[], int n, int m, struct Course courses[]) {
for (int i = 0; i < m; i++) {
strcpy(courses[i].name, "科目");
courses[i].name[5] = i + '1';
courses[i].name[6] = '\0';
courses[i].totalScore = 0;
for (int j = 0; j < n; j++) {
courses[i].totalScore += students[j].score[i];
}
courses[i].avgScore = courses[i].totalScore / n;
}
}
// 计算每个学生的总分和平均分
void calcStudentScore(struct Student students[], int n, int m) {
for (int i = 0; i < n; i++) {
students[i].totalScore = 0;
for (int j = 0; j < m; j++) {
students[i].totalScore += students[i].score[j];
}
students[i].avgScore = students[i].totalScore / m;
}
}
// 按每个学生的总分由高到低排出名次表
void sortStudentByTotalScore(struct Student students[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (students[i].totalScore < students[j].totalScore) {
struct Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
// 按每个学生的总分由低到高排出名次表
void sortStudentByTotalScoreReverse(struct Student students[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (students[i].totalScore > students[j].totalScore) {
struct Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
// 按学号由小到大排出成绩表
void sortStudentById(struct Student students[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (strcmp(students[i].id, students[j].id) > 0) {
struct Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
// 按姓名的字典顺序排出成绩表
void sortStudentByName(struct Student students[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (strcmp(students[i].name, students[j].name) > 0) {
struct Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
// 按学号查询学生排名及其各科考试成绩
void searchStudentById(struct Student students[], int n, char target[]) {
int rank = -1;
for (int i = 0; i < n; i++) {
if (strcmp(students[i].id, target) == 0) {
rank = i + 1;
printf("%d\t%s\t", rank, students[i].name);
for (int j = 0; j < 6; j++) {
printf("%.2f\t", students[i].score[j]);
}
printf("%.2f\t%.2f\n", students[i].totalScore, students[i].avgScore);
return;
}
}
printf("未找到学号为%s的学生\n", target);
}
// 按姓名查询学生排名及其各科考试成绩
void searchStudentByName(struct Student students[], int n, char target[]) {
int rank = -1;
for (int i = 0; i < n; i++) {
if (strcmp(students[i].name, target) == 0) {
rank = i + 1;
printf("%d\t%s\t", rank, students[i].id);
for (int j = 0; j < 6; j++) {
printf("%.2f\t", students[i].score[j]);
}
printf("%.2f\t%.2f\n", students[i].totalScore, students[i].avgScore);
return;
}
}
printf("未找到姓名为%s的学生\n", target);
}
```
阅读全文
相关推荐
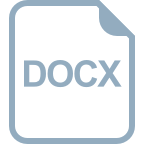
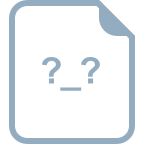















