TensorFlow手写体数字识别代码
时间: 2023-09-20 12:06:18 浏览: 157
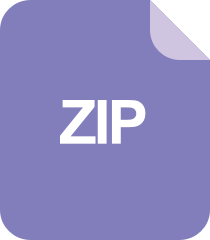
手写体数字识别(Python+TensorFlow)

### 回答1:
可以提供 TensorFlow 手写体数字识别的代码,以下是一个简单的示例:
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
# 加载数据集
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
# 定义输入和输出
x = tf.placeholder(tf.float32, [None, 784])
y_ = tf.placeholder(tf.float32, [None, 10])
# 定义模型
W = tf.Variable(tf.zeros([784, 10]))
b = tf.Variable(tf.zeros([10]))
y = tf.nn.softmax(tf.matmul(x, W) + b)
# 定义损失函数和优化器
cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.log(y), reduction_indices=[1]))
train_step = tf.train.GradientDescentOptimizer(.5).minimize(cross_entropy)
# 训练模型
sess = tf.InteractiveSession()
tf.global_variables_initializer().run()
for i in range(100):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y_: batch_ys})
# 评估模型
correct_prediction = tf.equal(tf.argmax(y,1), tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
print(sess.run(accuracy, feed_dict={x: mnist.test.images, y_: mnist.test.labels}))
```
这段代码使用 TensorFlow 实现了手写体数字识别,使用的是 MNIST 数据集。训练过程中使用了梯度下降优化器,最终输出了模型在测试集上的准确率。
### 回答2:
TensorFlow是一种用于构建、训练和部署机器学习模型的开源框架。手写体数字识别是机器学习中常见的问题之一,也是TensorFlow的经典示例之一。
在TensorFlow中,手写体数字识别可以通过卷积神经网络(CNN)实现。以下是一个简单的手写体数字识别的代码示例:
1. 导入必要的库和模块
```python
import tensorflow as tf
from tensorflow.keras import layers
```
2. 加载手写体数字数据集
```python
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
```
3. 数据预处理
```python
train_images = train_images.reshape(-1, 28, 28, 1)
train_images = train_images / 255.0
test_images = test_images.reshape(-1, 28, 28, 1)
test_images = test_images / 255.0
```
4. 构建卷积神经网络模型
```python
model = tf.keras.Sequential()
model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Flatten())
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(10, activation='softmax'))
```
5. 编译和训练模型
```python
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels))
```
6. 评估模型性能
```python
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
```
这是一个简单的手写体数字识别代码示例,通过TensorFlow的卷积神经网络模型,可以训练并评估手写体数字识别模型的准确性。这个示例可以作为入门学习 TensorFlow 和卷积神经网络的起点。
### 回答3:
TensorFlow手写体数字识别的代码主要包含以下几个步骤:
1. 导入必要的库和模块:首先需要导入TensorFlow库和其他必要的辅助库,例如numpy和matplotlib。
2. 数据准备:从MNIST数据库中加载手写数字图像数据集,并进行预处理。预处理过程包括将图像转化为灰度图像、将像素值进行归一化、将标签进行one-hot编码等。
3. 构建模型:定义神经网络模型的结构和参数。可以选择使用卷积神经网络(CNN)或全连接神经网络(FCN)等。根据模型的复杂程度和准确率要求,可以灵活地调整网络的层数和大小。
4. 模型训练:使用训练集数据对模型进行训练。通过传入训练集图像和对应的标签,计算模型的损失函数,并通过反向传播算法更新模型的参数,使得模型逐渐收敛到最优解。
5. 模型评估:使用测试集数据对训练好的模型进行评估。计算模型在测试集上的准确率或其他性能指标,以评估模型的性能。
6. 预测与可视化:使用训练好的模型对新的手写数字图像进行预测,并将预测结果进行可视化展示。
TensorFlow提供了丰富的工具和函数,能够有效地实现手写体数字识别。以上是一个基本的框架,具体的实现方式可以根据需求和任务的复杂度进行调整和扩展。使用TensorFlow编写手写体数字识别代码,可以在图像分类和识别任务中取得较高的准确率和性能。
阅读全文
相关推荐
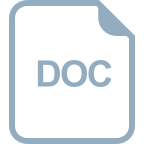
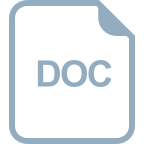

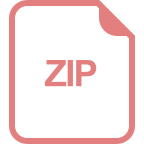
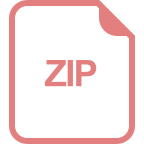

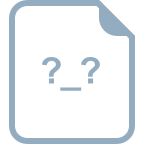
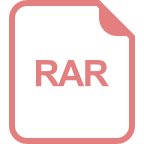
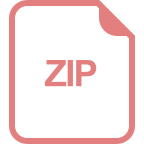
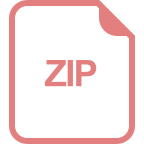
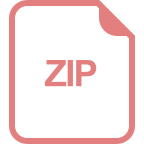
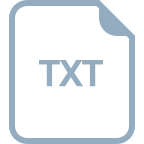
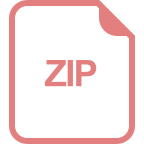
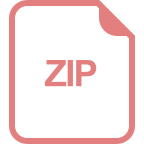
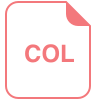

