二叉树遍历的完整代码c++语言版
时间: 2023-10-27 15:46:28 浏览: 78
#include<stdio.h>
#include<stdlib.h>
//定义二叉树结构体
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
//前序遍历
void preorderTraversal(struct TreeNode* root){
if(root==NULL)
return;
printf("%d ",root->val);
preorderTraversal(root->left);
preorderTraversal(root->right);
}
//中序遍历
void inorderTraversal(struct TreeNode* root){
if(root==NULL)
return;
inorderTraversal(root->left);
printf("%d ",root->val);
inorderTraversal(root->right);
}
//后序遍历
void postorderTraversal(struct TreeNode* root){
if(root==NULL)
return;
postorderTraversal(root->left);
postorderTraversal(root->right);
printf("%d ",root->val);
}
//创建节点
struct TreeNode* createNode(int val){
struct TreeNode* node=(struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val=val;
node->left=NULL;
node->right=NULL;
return node;
}
int main(){
struct TreeNode* root=createNode(1);
root->left=createNode(2);
root->right=createNode(3);
root->left->left=createNode(4);
root->left->right=createNode(5);
root->right->left=createNode(6);
root->right->right=createNode(7);
printf("前序遍历结果:");
preorderTraversal(root);
printf("\n中序遍历结果:");
inorderTraversal(root);
printf("\n后序遍历结果:");
postorderTraversal(root);
return 0;
}
阅读全文
相关推荐
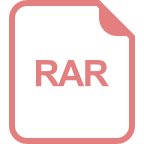



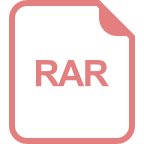
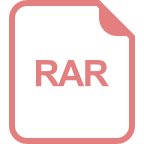






