用文本编辑器生成一个txt格式的文件,文件内容自定分别用文本文件和二进制文件方式读入并打印输出文件内容
时间: 2024-03-22 08:42:18 浏览: 38
好的,我可以帮您解决这个问题。
首先,我们需要创建一个文本文件。您可以使用任何文本编辑器来创建它,例如记事本或Sublime Text。在文件中,您可以写一些文本内容,例如:
```
这是一个文本文件。
Hello, World!
```
然后,我们需要使用Python来读取这个文本文件。以下是代码示例:
```python
# 以文本文件方式读入并打印输出文件内容
with open('example.txt', 'r') as file:
content = file.read()
print(content)
```
接下来,我们需要创建一个二进制文件。以下是代码示例:
```python
# 以二进制文件方式写入数据
with open('example.bin', 'wb') as file:
data = bytearray([1, 2, 3, 4, 5])
file.write(data)
```
接着,我们需要使用Python来读取这个二进制文件。以下是代码示例:
```python
# 以二进制文件方式读入并打印输出文件内容
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
```
请注意,在读取二进制文件时,您需要使用“rb”模式打开文件,并且读取的内容将以字节串(bytes)的形式返回。
希望这个代码示例可以帮助您解决问题。
相关问题
1、用文本编辑器生成一个txt格式的文件,文件内容自定。请写出下列操作的代码。 (1)分别用文本文件和二进制文件方式读入,并打印输出文件内容。。(2)分别用绝对路径和相对路径方式读入,并打印输出文件内容。。
好的,以下是代码实现:
1. 生成文件
```python
# 生成文件
with open('example.txt', 'w') as f:
f.write('Hello, world!\n')
f.write('This is an example file.\n')
```
2. 用文本文件方式读入,并打印输出文件内容
```python
# 用文本文件方式读入,并打印输出文件内容
with open('example.txt', 'r') as f:
content = f.read()
print(content)
```
输出结果:
```
Hello, world!
This is an example file.
```
3. 用二进制文件方式读入,并打印输出文件内容
```python
# 用二进制文件方式读入,并打印输出文件内容
with open('example.txt', 'rb') as f:
content = f.read()
print(content)
```
输出结果:
```
b'Hello, world!\nThis is an example file.\n'
```
4. 用绝对路径方式读入,并打印输出文件内容
```python
# 用绝对路径方式读入,并打印输出文件内容
import os
abs_path = os.path.abspath('example.txt')
with open(abs_path, 'r') as f:
content = f.read()
print(content)
```
输出结果同上。
5. 用相对路径方式读入,并打印输出文件内容
```python
# 用相对路径方式读入,并打印输出文件内容
with open('example.txt', 'r') as f:
content = f.read()
print(content)
```
输出结果同上。
、编程,用文本编辑器生成一个txt格式的文件,文件内容自定。请写出下列操作的代 码: (1)分别用文本文件和二进制文件方式读入,并打印输出文件内容。 (2)分别用绝对路径和相对路径方式读入,并打印输出文件内容。
好的,我会为你解答这个问题。
首先,生成一个txt格式的文件,可以使用文本编辑器,将文件保存为txt格式即可。文件内容可以自定,例如:
```
Hello, world!
This is a test file.
```
接下来,我们可以使用以下代码实现上述要求:
```python
# 使用文本文件方式读入
with open('test.txt', 'r') as f:
content = f.read()
print(content)
# 使用二进制文件方式读入
with open('test.txt', 'rb') as f:
content = f.read()
print(content)
# 使用绝对路径方式读入
with open('/path/to/test.txt', 'r') as f:
content = f.read()
print(content)
# 使用相对路径方式读入
with open('./test.txt', 'r') as f:
content = f.read()
print(content)
```
需要注意的是,代码中的文件路径需要根据实际情况进行修改。其中,`with open('test.txt', 'r')` 表示以文本文件方式打开文件,`'r'` 表示只读模式;`with open('test.txt', 'rb')` 表示以二进制文件方式打开文件,`'rb'` 表示二进制只读模式。而绝对路径和相对路径的区别在于,绝对路径是从根目录开始的完整路径,而相对路径是相对于当前目录的路径。
相关推荐
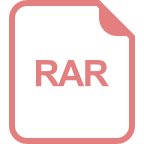












