使用c语言写一个学生管理系统
时间: 2023-08-31 21:32:38 浏览: 134
### 回答1:
在写一个学生管理系统时, 首先需要考虑使用哪些数据结构来存储学生信息, 例如可以使用结构体来存储每个学生的姓名, 学号, 成绩等信息. 然后需要考虑实现哪些功能, 例如可以实现增加学生, 删除学生, 查询学生, 修改学生信息等.
下面是一个示例代码:
```
#include<stdio.h>
#include<string.h>
struct student {
char name[20];
char id[20];
float score;
};
void addStudent(struct student stu[], int n) {
for (int i = 0; i < n; i++) {
printf("请输入第%d个学生的姓名: ", i+1);
scanf("%s", stu[i].name);
printf("请输入第%d个学生的学号: ", i+1);
scanf("%s", stu[i].id);
printf("请输入第%d个学生的成绩: ", i+1);
scanf("%f", &stu[i].score);
}
}
void deleteStudent(struct student stu[], int n, char* id) {
for (int i = 0; i < n; i++) {
if (strcmp(stu[i].id, id) == 0) {
for (int j = i; j < n-1; j++) {
stu[j] = stu[j+1];
}
n--;
break;
}
}
}
void searchStudent(struct student stu[], int n, char* id) {
for (int i = 0; i < n; i++) {
if (strcmp(stu[i].id, id) == 0) {
printf("姓名: %s\n", stu[i].name);
printf("学号: %s\n", stu[i].id);
printf("成绩: %.2f\n", stu[i].score);
break;
}
}
}
void updateStudent(struct student stu[], int n, char* id) {
for (int i = 0; i < n; i++) {
if (strcmp(stu[i].id
### 回答2:
学生管理系统是一个用来管理学生信息、成绩等相关数据的系统。使用C语言编写一个学生管理系统,可以通过以下步骤实现:
第一步:定义学生信息结构体
首先,定义一个结构体用于存储学生的信息,包括学号、姓名、性别、年龄、成绩等字段。
typedef struct {
int id; // 学号
char name[20]; // 姓名
char gender; // 性别
int age; // 年龄
float score; // 成绩
} Student;
第二步:实现学生信息的录入和显示
通过用户输入的方式,将学生的信息录入到一个数组中。同时,可以实现通过学号、姓名等方式查询学生信息,并将查询结果进行显示。
第三步:实现学生成绩的统计功能
可以实现对学生的成绩进行统计,包括计算总分、平均分、最高分、最低分等。
第四步:实现学生信息的修改和删除
可以通过学号或姓名找到要修改或删除的学生信息,并进行修改或删除操作。
第五步:实现学生信息的存储与读取
可以将学生的信息存储到文件中,以便下次使用时进行读取。
以上是一个简化版的学生管理系统的实现思路,通过C语言的基本逻辑、数据结构和文件操作等功能,可以完成一个基本的学生管理系统。当然,实际的学生管理系统可能还需要考虑更多的功能和细节,并进行更加完善的设计和实现。
阅读全文
相关推荐




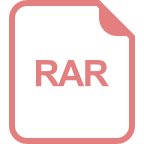
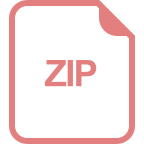


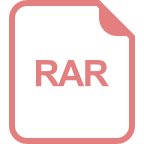



