<OTA_InventoryCheckRS TimeStamp=\"1629879848173\" Version=\"1.000\" EchoToken=\"18029461-194a-4c7e-b372-6eec346cebd4\"> <HotelResult> <HotelID>10000007</HotelID> <HotelProducts> <HotelProduct> <!--只有支持30分钟免费取消的酒店,才需要返回该节点--> <FreeCancelable FreeCancelableVal=\"true"\ /> <RoomTypes> <RoomType RoomTypeCode=\"2106\"/> </RoomTypes> <RatePlans> <RatePlan RatePlanCode=\"302-4529\"/> </RatePlans> <Prices> <Price> <Base EffectDate=\"2021-08-25\" SalePrice=\"515.2\" BasePrice=\"515.2\" Allotment=\"53\" DcOverSaleAllotment=\"53\" DcAddAllotment=\"-1\" DcContractAllotment=\"-1\" DcBuyOutAllotment=\"-1\"/> </Price> </Prices> </HotelProduct> </HotelProducts> </HotelResult> </OTA_InventoryCheckRS>转换为.net core模型
时间: 2024-02-12 08:03:41 浏览: 138
以下是将OTA_InventoryCheckRS转换为.NET Core模型的示例代码:
```csharp
using System.Collections.Generic;
using System.Xml.Serialization;
[XmlRoot(ElementName = "FreeCancelable")]
public class FreeCancelable
{
[XmlAttribute(AttributeName = "FreeCancelableVal")]
public string FreeCancelableVal { get; set; }
}
[XmlRoot(ElementName = "RoomType")]
public class RoomType
{
[XmlAttribute(AttributeName = "RoomTypeCode")]
public string RoomTypeCode { get; set; }
}
[XmlRoot(ElementName = "RatePlan")]
public class RatePlan
{
[XmlAttribute(AttributeName = "RatePlanCode")]
public string RatePlanCode { get; set; }
}
[XmlRoot(ElementName = "Base")]
public class Base
{
[XmlAttribute(AttributeName = "EffectDate")]
public string EffectDate { get; set; }
[XmlAttribute(AttributeName = "SalePrice")]
public string SalePrice { get; set; }
[XmlAttribute(AttributeName = "BasePrice")]
public string BasePrice { get; set; }
[XmlAttribute(AttributeName = "Allotment")]
public string Allotment { get; set; }
[XmlAttribute(AttributeName = "DcOverSaleAllotment")]
public string DcOverSaleAllotment { get; set; }
[XmlAttribute(AttributeName = "DcAddAllotment")]
public string DcAddAllotment { get; set; }
[XmlAttribute(AttributeName = "DcContractAllotment")]
public string DcContractAllotment { get; set; }
[XmlAttribute(AttributeName = "DcBuyOutAllotment")]
public string DcBuyOutAllotment { get; set; }
}
[XmlRoot(ElementName = "Price")]
public class Price
{
[XmlElement(ElementName = "Base")]
public Base Base { get; set; }
}
[XmlRoot(ElementName = "HotelProduct")]
public class HotelProduct
{
[XmlElement(ElementName = "FreeCancelable")]
public FreeCancelable FreeCancelable { get; set; }
[XmlElement(ElementName = "RoomTypes")]
public RoomTypes RoomTypes { get; set; }
[XmlElement(ElementName = "RatePlans")]
public RatePlans RatePlans { get; set; }
[XmlElement(ElementName = "Prices")]
public Prices Prices { get; set; }
}
[XmlRoot(ElementName = "RoomTypes")]
public class RoomTypes
{
[XmlElement(ElementName = "RoomType")]
public RoomType RoomType { get; set; }
}
[XmlRoot(ElementName = "RatePlans")]
public class RatePlans
{
[XmlElement(ElementName = "RatePlan")]
public RatePlan RatePlan { get; set; }
}
[XmlRoot(ElementName = "Prices")]
public class Prices
{
[XmlElement(ElementName = "Price")]
public Price Price { get; set; }
}
[XmlRoot(ElementName = "HotelResult")]
public class HotelResult
{
[XmlElement(ElementName = "HotelID")]
public string HotelID { get; set; }
[XmlElement(ElementName = "HotelProducts")]
public HotelProducts HotelProducts { get; set; }
}
[XmlRoot(ElementName = "HotelProducts")]
public class HotelProducts
{
[XmlElement(ElementName = "HotelProduct")]
public HotelProduct HotelProduct { get; set; }
}
[XmlRoot(ElementName = "OTA_InventoryCheckRS")]
public class OTA_InventoryCheckRS
{
[XmlElement(ElementName = "HotelResult")]
public HotelResult HotelResult { get; set; }
[XmlAttribute(AttributeName = "TimeStamp")]
public string TimeStamp { get; set; }
[XmlAttribute(AttributeName = "Version")]
public string Version { get; set; }
[XmlAttribute(AttributeName = "EchoToken")]
public string EchoToken { get; set; }
}
```
你可以使用XmlSerializer来反序列化XML字符串到OTA_InventoryCheckRS类的实例中,示例代码如下:
```csharp
string xmlString = "<OTA_InventoryCheckRS TimeStamp=\"1629879848173\" Version=\"1.000\" EchoToken=\"18029461-194a-4c7e-b372-6eec346cebd4\"><HotelResult><HotelID>10000007</HotelID><HotelProducts><HotelProduct><FreeCancelable FreeCancelableVal=\"true\"/><RoomTypes><RoomType RoomTypeCode=\"2106\"/></RoomTypes><RatePlans><RatePlan RatePlanCode=\"302-4529\"/></RatePlans><Prices><Price><Base EffectDate=\"2021-08-25\" SalePrice=\"515.2\" BasePrice=\"515.2\" Allotment=\"53\" DcOverSaleAllotment=\"53\" DcAddAllotment=\"-1\" DcContractAllotment=\"-1\" DcBuyOutAllotment=\"-1\"/></Price></Prices></HotelProduct></HotelProducts></HotelResult></OTA_InventoryCheckRS>";
XmlSerializer serializer = new XmlSerializer(typeof(OTA_InventoryCheckRS));
using (var reader = new StringReader(xmlString))
{
OTA_InventoryCheckRS result = (OTA_InventoryCheckRS)serializer.Deserialize(reader);
// result即为反序列化后的OTA_InventoryCheckRS实例
}
```
阅读全文
相关推荐







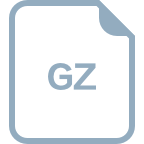


