rrt路径规划matlab代码
时间: 2023-11-16 11:07:31 浏览: 37
由于RRt路径规划算法涉及到很多细节,代码也较为复杂,因此在此提供一个参考链接:https://github.com/AtsushiSakai/MATLABRobotics/tree/master/PathPlanning/RRTStar
该代码实现了RRT*、RRT-Connect和Bi-Directional RRT*三种不同的RRt路径规划算法,包括生成树的构建、路径搜索、障碍物检测等多个模块。可以通过该代码学习RRt路径规划算法的实现细节,也可以在此基础上进行扩展和改进。
相关问题
机械臂RRT路径规划matlab
以下是一个简单的机械臂RRT路径规划的MATLAB代码示例:
```matlab
function path = rrtPathPlanning(start, goal, obstacles, step_size, max_iterations)
tree = Node(start);
for iter = 1:max_iterations
if rand() < 0.5
random_config = rand(size(start)) * 2 * pi - pi; % 随机生成关节配置
else
random_config = goal; % 以目标配置为随机点
end
nearest_node = nearestNeighbor(tree, random_config); % 寻找最近的节点
new_config = stepFromTo(nearest_node.configuration, random_config, step_size); % 在最近节点和随机点之间前进一步
if isValidConfiguration(new_config, obstacles) % 检查新配置是否有效
new_node = Node(new_config, nearest_node);
tree = [tree new_node]; % 将新节点添加到树中
if norm(new_config - goal) < step_size % 如果新配置接近目标配置
path = getPath(tree, new_node); % 获取路径
return;
end
end
end
path = []; % 无法找到路径
end
function nearest_node = nearestNeighbor(tree, config)
distances = arrayfun(@(node) norm(node.configuration - config), tree); % 计算每个节点与给定配置的距离
[~, index] = min(distances); % 找到最小距离的节点索引
nearest_node = tree(index);
end
function new_config = stepFromTo(start_config, end_config, step_size)
direction = end_config - start_config;
distance = norm(direction);
if distance <= step_size
new_config = end_config;
else
unit_direction = direction / distance;
new_config = start_config + step_size * unit_direction;
end
end
function valid = isValidConfiguration(config, obstacles)
% 检查配置是否与障碍物发生碰撞
% 返回true表示有效,false表示无效
end
function path = getPath(tree, end_node)
path = [];
current_node = end_node;
while ~isempty(current_node)
path = [current_node.configuration; path];
current_node = current_node.parent;
end
end
classdef Node
properties
configuration % 关节配置
parent % 父节点
end
methods
function obj = Node(config, parent)
obj.configuration = config;
obj.parent = parent;
end
end
end
```
以上代码是一个简单的使用RRT算法进行机械臂路径规划的示例。你可以根据实际情况进行修改和扩展,例如添加适当的碰撞检测和路径优化方法。
rrt路径规划算法matlab
以下是一个简单的RRT路径规划算法的MATLAB实现,仅供参考。
```matlab
% RRT路径规划算法
% 作者: Yijun Yuan (yyuan@mit.edu)
%
% 输入:
% start_pos: 起点坐标
% goal_pos: 终点坐标
% obstacle_list: 障碍物列表
% max_iter: 最大迭代次数
% step_size: 步长
% 输出:
% path: 路径坐标列表
function path = RRT(start_pos, goal_pos, obstacle_list, max_iter, step_size)
% 初始化树
tree = [start_pos, 0];
for i = 1:max_iter
% 随机生成一个点
rand_pos = [rand*10, rand*10];
% 找到距离随机点最近的树上节点
dist = sqrt((tree(:,1) - rand_pos(1)).^2 + (tree(:,2) - rand_pos(2)).^2);
[~, nearest_idx] = min(dist);
nearest_node = tree(nearest_idx,:);
% 计算朝向随机点的向量
dir_vec = rand_pos - nearest_node(1:2);
dir_vec = dir_vec / norm(dir_vec);
% 计算新节点位置
new_pos = nearest_node(1:2) + dir_vec * step_size;
% 如果新节点不在障碍物内,则加入树
if ~IsInObstacle(new_pos, obstacle_list)
new_node = [new_pos, nearest_idx];
tree = [tree; new_node];
% 如果新节点距离目标点小于一定阈值,则认为找到了一条路径
if norm(new_pos - goal_pos) < 0.5
path = BacktrackPath(tree, nearest_idx);
return
end
end
end
% 没有找到路径
path = [];
end
function path = BacktrackPath(tree, goal_idx)
% 回溯路径
path = tree(goal_idx, 1:2);
while goal_idx ~= 1
goal_idx = tree(goal_idx, 3);
path = [tree(goal_idx, 1:2); path];
end
end
function is_in = IsInObstacle(pos, obstacle_list)
% 判断点是否在障碍物内
is_in = false;
for i = 1:length(obstacle_list)
obs = obstacle_list{i};
if pos(1) >= obs(1) && pos(1) <= obs(3) && pos(2) >= obs(2) && pos(2) <= obs(4)
is_in = true;
break;
end
end
end
```
需要注意的是,此实现仅考虑二维平面,且障碍物为矩形。如果需要考虑更复杂的情况,需要对代码进行相应的修改。
相关推荐
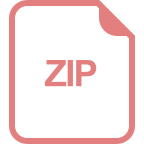
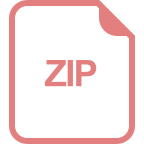
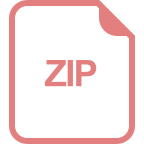












