python利用四点平行四边形坐标裁剪float32类型图像
时间: 2023-10-12 16:19:55 浏览: 67
在Python中,你可以使用OpenCV库来进行图像裁剪。以下是一个示例代码,其中包括了一个函数 `crop_image()`,该函数可以将图像裁剪为四边形区域:
```python
import cv2
import numpy as np
def crop_image(image, pts):
"""
:param image: ndarray, float32类型的图像
:param pts: ndarray, 形如[[x1,y1], [x2,y2], [x3,y3], [x4,y4]]的四点坐标数组
:return: ndarray, 裁剪后的图像
"""
# 将四点坐标转换为矩形
rect = cv2.minAreaRect(pts)
box = cv2.boxPoints(rect)
box = np.int0(box)
# 透视变换
width = int(rect[1][0])
height = int(rect[1][1])
src_pts = box.astype("float32")
dst_pts = np.array([[0, height-1],
[0, 0],
[width-1, 0],
[width-1, height-1]], dtype="float32")
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
cropped = cv2.warpPerspective(image, M, (width, height))
return cropped
```
使用该函数,你可以将一个四点平行四边形的区域裁剪出来。你需要提供一个 `ndarray` 类型的图像和四个坐标点,函数将返回裁剪后的图像。
示例代码:
```python
# 读取图像
image = cv2.imread('example.jpg').astype(np.float32)
# 四点坐标
pts = np.array([[50, 50], [300, 100], [200, 400], [20, 300]], dtype=np.float32)
# 裁剪图像
cropped = crop_image(image, pts)
# 显示原始图像和裁剪后的图像
cv2.imshow('Original Image', image.astype(np.uint8))
cv2.imshow('Cropped Image', cropped.astype(np.uint8))
cv2.waitKey(0)
```
在上面的示例中,我们使用 `cv2.imread()` 函数读取了一张图像,然后指定了一个四点坐标数组 `pts`,最后调用了 `crop_image()` 函数来裁剪图像。
相关推荐
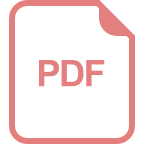
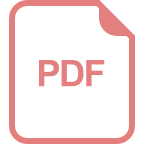














